.Net Core is getting more and more popular recently due to the flexibility to work and be hosted in different environments like Windows, Mac, or Linux. In this article, we will learn how to build a .Net MVC Core website in an N-tier project structure.
1. The Project Overview - What Are We Building?
We are building a simple .Net MVC Core website to support the requirements of a personal blog that allows users to add, edit information about themselves as well as to post, modify, or archive their articles in this website. This web application does not use external database.
Module 1: Home Page Requirements
- Display Welcome Message
- Display Navigation to About Me page
- Randomly display different Welcome Animated Images
Module 2: About Me Requirements
- Display the blog's owner information: Name, Biography, Work Experiences, Skills, Education, Portfolio, and Entry to their Blog
- Allow owner to add, edit, or delete their information
- Allow owner to upload profile image
Module 3: Blog Requirements
- Display all articles which are created by the owner
- Allow owner to add, edit, or archive their posted articles
- Allow users to search articles by keyword, filter by category, view top popular and favorite articles
Module 4: Contact Requirements
- Simply show owner contact information
General Requirements
- Owner should be able to login by their default password
- Add some animations to make the website nicer
- Dynamically created sitemap page for the website
- Dynamically injected json-ld for article page
2. What Are We Going To Use?
We have all the requirements for this web application. Now we have to decide the technologies we are going to use in order to build our .Net MVC Core application.
Tools:
- Visual Studio 2019
Server Side:
- Net Core 2.0: newer version can be used but since I'm hosting on GoDaddy, I will use Net Core 2.0.
- Entity Framework Core
- Automapper
Client Side:
- Bootstrap 4.3
- Summernote
- SyntaxHighlighter
3. Create an N-Tire Structured Project
We are building this web application in an N-Tire Structure which has Data, Business, and Web layers.
Step 1: Create a Blank Solution
Open Visual Studio 2019, search for Blank Solution, name the solution "Blog" and then Create:
Step 2: Create a Data Layer
Right click on the Blog solution, Add New Project and Select Class Library (.Net Core). Name the Project "Blog.DAL"
Step 3: Create a Business Layer
Right click on the Blog solution, Add New Project and Select Class Library (.Net Core). Name the Project "Blog.BAL"
Step 4: Create a Web Layer
Right click on the Blog solution, Add New Project and Select ASP.Net Core Web Application. Name the Project "Blog.Web"
Select ASP.Net Core 2.0 and Web Application (Model-View-Controller):
Step 5: Connecting the 3 projects
Add reference to connect the 3 projects in below order:
Blog.DAL >> Blog.BAL >> Blog.Web
To add Project Reference, right click on Project in Solution Explorer, select Add > Project Reference...:
4. Build the .Net MVC Core Application
Ok, let's actually write the code to build the website. We will go to each project and do what we need to do for each:
- Blog.DAL: This project consists of all entity classes for the application.
- Blog.BAL: This project consists of all business logic to manipulate the data getting from Blog.DAL project.
- Blog.Web: This project is to build the front-end part of the application based on the data set returned from the Blog.BAL project.
Step 1: Build Blog.DAL project
Install below package from Manage NuGet Packages:
- Newtonsoft.Json (12.0.3)
The Blog.DAL project has below structure:
1. In Entities folder, create below classes:
BaseEntity.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | using System; namespace Blog.DAL.Entities { public abstract class BaseEntity { public BaseEntity() { this .ID = Guid.NewGuid(); this .CreatedOn = DateTime.UtcNow; this .UpdatedOn = DateTime.UtcNow; this .IsActive = true ; this .IsVisible = true ; } public Guid ID { get ; set ; } public DateTime CreatedOn { get ; set ; } public DateTime UpdatedOn { get ; set ; } public bool IsActive { get ; set ; } public bool IsVisible { get ; set ; } } } |
Education.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | namespace Blog.DAL.Entities { public class Education : BaseEntity { public string School { get ; set ; } public string Major { get ; set ; } public string Location { get ; set ; } public string Duration { get ; set ; } public string Items { get ; set ; } } } |
Experience.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | namespace Blog.DAL.Entities { public class Experience : BaseEntity { public string Title { get ; set ; } public string Company { get ; set ; } public string Location { get ; set ; } public string Duration { get ; set ; } public string Items { get ; set ; } } } |
LdJsonObject.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 | using Newtonsoft.Json; using System.Collections.Generic; namespace Blog.DAL.Entities { public class ArticleLdJson { public string type { get ; set ; } = "NewsArticle" ; public MainEntityOfPage mainEntityOfPage { get ; set ; } public string headline { get ; set ; } public Image image { get ; set ; } public string datePublished { get ; set ; } public string dateModified { get ; set ; } public Author author { get ; set ; } public Publisher publisher { get ; set ; } public string description { get ; set ; } public override string ToString() { return JsonConvert.SerializeObject( this ) .Replace( "context" , "@context" ) .Replace( "type" , "@type" ) .Replace( "id\"" , "@id\"" ); } } public class MainEntityOfPage { public string type { get ; set ; } = "WebPage" ; public string id { get ; set ; } } public class Image { public string type { get ; set ; } = "ImageObject" ; public string url { get ; set ; } public string width { get ; set ; } = "700px" ; public string height { get ; set ; } = "400px" ; } public class Author { public string type { get ; set ; } = "Person" ; public string name { get ; set ; } } public class Publisher { public string type { get ; set ; } = "Organization" ; public string name { get ; set ; } = "Lucasology" ; public Logo logo { get ; set ; } = new Logo(); } public class Logo { public string type { get ; set ; } = "ImageObject" ; public string url { get ; set ; } public string width { get ; set ; } = "512px" ; public string height { get ; set ; } = "512px" ; } public class OrganizationLdJson { public string type { get ; set ; } = "Organization" ; public string name { get ; set ; } public string url { get ; set ; } public string logo { get ; set ; } public string foundingDate { get ; set ; } public List<Author> founders { get ; set ; } public List< string > sameAs { get ; set ; } public override string ToString() { return JsonConvert.SerializeObject( this ) .Replace( "context" , "@context" ) .Replace( "type" , "@type" ) .Replace( "id" , "@id" ); } } public class BreadcrumLdJson { public string type { get ; set ; } = "BreadcrumbList" ; public List<BreadcrumElement> itemListElement { get ; set ; } = new List<BreadcrumElement>(); public override string ToString() { return JsonConvert.SerializeObject( this ) .Replace( "context" , "@context" ) .Replace( "type" , "@type" ) .Replace( "id" , "@id" ); } } public class BreadcrumElement { public string type { get ; set ; } = "ListItem" ; public int position { get ; set ; } public string name { get ; set ; } public string item { get ; set ; } } public class CarouselLdJson { public string type { get ; set ; } = "ItemList" ; public List<CarouselElement> itemListElement { get ; set ; } = new List<CarouselElement>(); public override string ToString() { return JsonConvert.SerializeObject( this ) .Replace( "context" , "@context" ) .Replace( "type" , "@type" ) .Replace( "id" , "@id" ); } } public class CarouselElement { public string type { get ; set ; } = "ListItem" ; public int position { get ; set ; } public string url { get ; set ; } } } |
Post.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 | using System; using System.Collections.Generic; namespace Blog.DAL.Entities { public class Post : BaseEntity { public string Title { get ; set ; } public string Category { get ; set ; } public string Series { get ; set ; } public string AuthorName { get ; set ; } public int LikeCount { get ; set ; } public int ViewCount { get ; set ; } public string Thumbnail { get ; set ; } public string Content { get ; set ; } public string Preview { get ; set ; } public string Tags { get ; set ; } public List< string > Contents { get ; set ; } public DateTime PublishedDate { get ; set ; } } } |
Project.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 | using System.Collections.Generic; namespace Blog.DAL.Entities { public class Project : BaseEntity { public string Name { get ; set ; } public string Description { get ; set ; } public string Image { get ; set ; } public ProjectLinks Links { get ; set ; } } public class ProjectLinks { public ProjectLinks() { Links = new List<ProjectLink>(); } public List<ProjectLink> Links { get ; set ; } } public class ProjectLink : BaseEntity { public string Link { get ; set ; } public string LinkName { get ; set ; } } } |
SitemapUrl.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 | using System; using System.Collections.Generic; using System.Linq; using System.Xml.Linq; namespace Blog.DAL.Entities { public enum ChangeFrequency { Always, Hourly, Daily, Weekly, Yearly, Never } public class SitemapUrl { public string Url { get ; set ; } public DateTime? Modified { get ; set ; } public ChangeFrequency? ChangeFrequency { get ; set ; } public double ? Priority { get ; set ; } } public class SitemapBuilder { private List<SitemapUrl> _urls; public SitemapBuilder() { _urls = new List<SitemapUrl>(); } public void AddUrl( string url, DateTime? modified = null , ChangeFrequency? changeFrequency = null , double ? priority = null ) { _urls.Add( new SitemapUrl() { Url = url, Modified = modified, ChangeFrequency = changeFrequency, Priority = priority }); } public override string ToString() { var sitemap = new XDocument( new XDeclaration( "1.0" , "utf-8" , "yes" ), new XElement(NS + "urlset" , from item in _urls select CreateItemElement(item) )); return sitemap.ToString(); } private XElement CreateItemElement(SitemapUrl url) { XElement itemElement = new XElement(NS + "url" , new XElement(NS + "loc" , url.Url)); if (url.Modified.HasValue) { itemElement.Add( new XElement(NS + "lastmod" , url.Modified.Value.ToString( "yyyy-MM-dd" ))); } if (url.ChangeFrequency.HasValue) { itemElement.Add( new XElement(NS + "changefreq" , url.ChangeFrequency.Value.ToString())); } if (url.Priority.HasValue) { itemElement.Add( new XElement(NS + "priority" , url.Priority.Value.ToString( "N1" ))); } return itemElement; } } } |
Skill.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 | namespace Blog.DAL.Entities { public class Skill : BaseEntity { public string Category { get ; set ; } public string Name { get ; set ; } public int ? StartYear { get ; set ; } public double ? Rating { get ; set ; } } } |
User.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 | using System.Collections.Generic; namespace Blog.DAL.Entities { public class User : BaseEntity { public string Name { get ; set ; } public string Summary { get ; set ; } public string ProfileImage { get ; set ; } public List< string > Achievements { get ; set ; } public List<Skill> Skills { get ; set ; } public List<Experience> Experiences { get ; set ; } public List<Education> Educations { get ; set ; } public List<Project> Projects { get ; set ; } } } |
Step 2: Build Blog.BAL project
Install below packages from Manage NuGet Packages:
- HtmlAgilityPack.NetCore (1.5.0.1)
- Microsoft.AspNetCore.Hosting (2.2.7)
- Microsoft.AspNetCore.Http (2.2.2)
- Microsoft.Extensions.Caching.Memory (2.0.2)
- Microsoft.Extensions.Configuration.Json (3.1.9)
- Newtonsoft.Json (12.0.3)
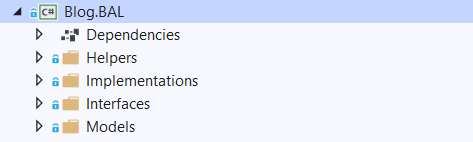
1. In Helpers folder, create below classes
AppContext.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 | using Microsoft.AspNetCore.Http; using Microsoft.Extensions.Caching.Memory; using System; using System.Net; using System.Threading; using System.Threading.Tasks; namespace Blog.BAL.Helpers { public static class MyAppContext { private static IHttpContextAccessor _httpContextAccessor; private static IMemoryCache _cache; public static void Configure(IHttpContextAccessor httpContextAccessor, IMemoryCache cache) { _httpContextAccessor = httpContextAccessor; _cache = cache; } public static IHttpContextAccessor Current => _httpContextAccessor; public static void SetupRefreshJob() { Action remove = _cache.Get( "Refresh" ) as Action; if (remove is Action) { _cache.Remove( "Refresh" ); remove.EndInvoke( null ); } //get the worker Action work = () => { while ( true ) { Thread.Sleep(60000); WebClient refresh = new WebClient(); try { } catch (Exception ex) { //Log Error here } finally { refresh.Dispose(); } } }; Task.Run(() => work.Invoke()); //add this job to cache _cache.Set( "Refresh" , work, new MemoryCacheEntryOptions() { AbsoluteExpiration = DateTimeOffset.MaxValue, SlidingExpiration = TimeSpan.FromDays(365), Priority = CacheItemPriority.Normal }); } } } |
MySession.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 | using Microsoft.AspNetCore.Http; using System; namespace Blog.BAL.Helpers { public static class MySession { public static void Set( this IHttpContextAccessor accessor, string key, string value, DateTime expiredDate) { var cookieOptions = new CookieOptions { HttpOnly = false , IsEssential = true , Expires = expiredDate }; accessor.HttpContext.Response.Cookies.Append(key, value, cookieOptions); } public static string Get( this IHttpContextAccessor accessor, string key) { string val = null ; if (accessor.HttpContext.Request.Cookies[key] != null ) { val = accessor.HttpContext.Request.Cookies[key]; } return val; } public static void Remove( this IHttpContextAccessor accessor, string key) { if (accessor.HttpContext.Request.Cookies[key] != null ) { accessor.HttpContext.Response.Cookies.Delete(key); } } } } |
PaginatedList.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 | using System; using System.Collections.Generic; using System.Linq; namespace Blog.BAL.Helpers { public class PaginatedList<T> : List<T> { public int PageIndex { get ; private set ; } public int TotalPages { get ; private set ; } public PaginatedList(List<T> items, int count, int pageIndex, int pageSize) { PageIndex = pageIndex; TotalPages = ( int )Math.Ceiling(count / ( double )pageSize); this .AddRange(items); } public bool HasPreviousPage { get { return (PageIndex > 1); } } public bool HasNextPage { get { return (PageIndex < TotalPages); } } public static PaginatedList<T> Create(List<T> source, int pageIndex, int pageSize) { var count = source.Count(); var items = source.Skip((pageIndex - 1) * pageSize).Take(pageSize).ToList(); return new PaginatedList<T>(items, count, pageIndex, pageSize); } } } |
Utility.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 | using Microsoft.AspNetCore.Http; using Microsoft.Extensions.Configuration; using System; using System.Collections.Generic; using System.Configuration; using System.IO; using System.Security.Cryptography; using System.Text; namespace Blog.BAL.Helpers { public class Utility { public static string GetValueByKey( string key) { IConfigurationSection appSetting = AppSetting(key); return appSetting.Value; } public static string EncryptPassword( string password, string salt) { using (var sha256 = SHA256.Create()) { var saltedPassword = string .Format( "{0}{1}" , salt, password); byte [] saltedPasswordAsBytes = Encoding.UTF8.GetBytes(saltedPassword); return Convert.ToBase64String(sha256.ComputeHash(saltedPasswordAsBytes)); } } private static IConfigurationSection AppSetting( string key) { var configBuilder = new ConfigurationBuilder(); var path = Path.Combine(Directory.GetCurrentDirectory(), "appsettings.json" ); configBuilder.AddJsonFile(path, false ); var root = configBuilder.Build(); var appSetting = root.GetSection(key); return appSetting; } public static int YearsOfExperience() { return DateTime.Now.Year - Convert.ToInt32(Utility.GetValueByKey( "StartYear" )); } public static double ? CalculateSkillRating( double ? startYear) { return (( double )DateTime.Now.Year - ( double )startYear) / ( double )Utility.YearsOfExperience(); } public static string GetUniqueFileName( string fileName) { fileName = Path.GetFileName(fileName); return Path.GetFileNameWithoutExtension(fileName) + "_" + Guid.NewGuid().ToString().Substring(0, 4) + Path.GetExtension(fileName); } public static string ConvertToBase64Image(IFormFile formFile) { string s = null ; using (var ms = new MemoryStream()) { formFile.CopyTo(ms); var fileBytes = ms.ToArray(); s = Convert.ToBase64String(fileBytes); } return s; } public static bool IsBase64( string base64String) { if ( string .IsNullOrEmpty(base64String) || base64String.Length % 4 != 0 || base64String.Contains( " " ) || base64String.Contains( "\t" ) || base64String.Contains( "\r" ) || base64String.Contains( "\n" )) return false ; try { Convert.FromBase64String(base64String); return true ; } catch { // Handle the exception } return false ; } public static string ConvertBase64ToImage( string base64Img, string path) { string profileImageName = Guid.NewGuid() + ".jpg" ; //Convert Base64 Encoded string to Byte Array. byte [] imageBytes = Convert.FromBase64String(base64Img); //Save the Byte Array as Image File. var imgFilePath = Path.Combine(path, profileImageName); File.WriteAllBytes(imgFilePath, imageBytes); return profileImageName; } } } |
2. In Implementation folder, create below classes
AchievementManager.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 | using Blog.BAL.Interfaces; using Microsoft.AspNetCore.Http; using Newtonsoft.Json; using System.Collections.Generic; using System.IO; using Microsoft.AspNetCore.Hosting; namespace Blog.BAL.Implementations { public class AchievementManager : IAchievementManager { private readonly string contentRootPath = Directory.GetCurrentDirectory(); private readonly IHttpContextAccessor _httpContextAccessor; private readonly IHostingEnvironment _hostingEnvironment; public AchievementManager(IHttpContextAccessor httpContextAccessor, IHostingEnvironment hostingEnvironment) { _httpContextAccessor = httpContextAccessor; _hostingEnvironment = hostingEnvironment; } #region private methods private List< string > ConvertToDTO(List< string > model) { List< string > achievements = new List< string >(); foreach (var s in model) { achievements.Add(s); } return achievements; } #endregion #region public methods public void EditAchievements(List< string > model) { string filePath = $ "{contentRootPath}\\Database\\Achievements.json" ; File.WriteAllText(filePath, JsonConvert.SerializeObject(ConvertToDTO(model))); } public List< string > GetAchievements() { string filePath = $ "{contentRootPath}\\Database\\Achievements.json" ; List< string > achievements = new List< string >(); if (File.Exists(filePath)) achievements = JsonConvert.DeserializeObject<List< string >>(System.IO.File.ReadAllText(filePath)); return achievements; } #endregion } } |
BlogManager.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 | using Blog.BAL.Interfaces; using Blog.BAL.Models; using Blog.BAL.Helpers; using Blog.DAL.Entities; using Newtonsoft.Json; using System; using System.Collections.Generic; using System.IO; using Microsoft.AspNetCore.Hosting; using System.Linq; using System.Net; using HtmlAgilityPack; using System.Text.RegularExpressions; using System.Web; namespace Blog.BAL.Implementations { public class BlogManager : IBlogManager { private readonly string contentRootPath = Directory.GetCurrentDirectory(); private readonly IHostingEnvironment _hostingEnvironment; public BlogManager(IHostingEnvironment hostingEnvironment) { _hostingEnvironment = hostingEnvironment; } #region public methods public void Create(PostViewModel model) { string filePath = $ "{contentRootPath}\\Database\\Posts.json" ; ProcessPost(model); List<Post> posts = new List<Post>(); if (File.Exists(filePath)) posts = JsonConvert.DeserializeObject<List<Post>>(System.IO.File.ReadAllText(filePath)); var newPost = ConvertToDTO(model); posts.Add(ConvertToDTO(model)); File.WriteAllText(filePath, JsonConvert.SerializeObject(posts)); } #endregion #region public methods public void Edit(PostViewModel model) { string filePath = $ "{contentRootPath}\\Database\\Posts.json" ; Regex tagRegex = new Regex( @"<[^>]+>" ); if (tagRegex.IsMatch(model.Content)) ProcessPost(model); List<Post> posts = new List<Post>(); if (File.Exists(filePath)) posts = JsonConvert.DeserializeObject<List<Post>>(System.IO.File.ReadAllText(filePath)); var index = posts.FindIndex(p => p.ID == model.ID); model.Thumbnail = model.Thumbnail == null ? posts[index].Thumbnail : model.Thumbnail; posts[index] = ConvertToDTO(model); posts[index].UpdatedOn = DateTime.UtcNow; File.WriteAllText(filePath, JsonConvert.SerializeObject(posts)); } public List<Post> GetPosts( int ? take) { string filePath = $ "{contentRootPath}\\Database\\Posts.json" ; List<Post> posts = new List<Post>(); if (File.Exists(filePath)) posts = JsonConvert.DeserializeObject<List<Post>>(System.IO.File.ReadAllText(filePath)) .Where(p => p.PublishedDate <= DateTime.UtcNow && p.IsActive) .OrderByDescending(p => p.UpdatedOn).ToList(); return take == null ? posts : posts.Take(( int )take).ToList(); } public List<Post> GetAllPosts( int ? take) { string filePath = $ "{contentRootPath}\\Database\\Posts.json" ; List<Post> posts = new List<Post>(); if (File.Exists(filePath)) posts = JsonConvert.DeserializeObject<List<Post>>(System.IO.File.ReadAllText(filePath)) .OrderByDescending(p => p.UpdatedOn).ToList(); return take == null ? posts : posts.Take(( int )take).ToList(); } public Post GetPostByID(Guid ID) { string filePath = $ "{contentRootPath}\\Database\\Posts.json" ; List<Post> posts = new List<Post>(); if (File.Exists(filePath)) posts = JsonConvert.DeserializeObject<List<Post>>(System.IO.File.ReadAllText(filePath)); return posts.Where(p => p.ID == ID).FirstOrDefault(); } public Post GetPostByUrl( string url) { string filePath = $ "{contentRootPath}\\Database\\Posts.json" ; List<Post> posts = new List<Post>(); if (File.Exists(filePath)) posts = JsonConvert.DeserializeObject<List<Post>>(System.IO.File.ReadAllText(filePath)); return string .IsNullOrEmpty(url)? null : posts.Where(p => p.Content.ToUpper() == HttpUtility.UrlDecode(url).ToUpper() && p.IsActive).FirstOrDefault(); } public Post GetPostByUrlForArchival( string url) { string filePath = $ "{contentRootPath}\\Database\\Posts.json" ; List<Post> posts = new List<Post>(); if (File.Exists(filePath)) posts = JsonConvert.DeserializeObject<List<Post>>(System.IO.File.ReadAllText(filePath)); return string .IsNullOrEmpty(url) ? null : posts.Where(p => p.Content.ToUpper() == HttpUtility.UrlDecode(url).ToUpper()).FirstOrDefault(); } public string GetContentByUrl( string url) { string filePath = $ "{contentRootPath}\\Blog\\{url}.html" ; string content = "" ; if (File.Exists(filePath)) content = File.ReadAllText(filePath); return content; } #endregion #region private methods private void ProcessPost(PostViewModel model) { //ProcessThumbnail if (model.FormFile != null ) { var uniqueFileName = Utility.GetUniqueFileName(model.FormFile.FileName); var uploads = Path.Combine(_hostingEnvironment.WebRootPath, "Uploads/BlogImages" ); var imgFilePath = Path.Combine(uploads, uniqueFileName); model.FormFile.CopyTo( new FileStream(imgFilePath, FileMode.Create)); model.Thumbnail = uniqueFileName; } //ProcessContentHtml ProcessContentHTML(model); //ProcessContent WriteHTML(model); } private void WriteHTML(PostViewModel model) { string uniqueTitle; //Make sure not create new html file while editing if (model.ID == Guid.Empty) uniqueTitle = Utility.GetUniqueFileName(model.Title); else uniqueTitle = this .GetPostByID(model.ID).Content; string filePath = $ "{contentRootPath}\\Blog\\" + uniqueTitle + ".html" ; File.WriteAllText(filePath, model.Content); model.Preview = Regex.Replace(model.Content, "<.*?>" , String.Empty); if (model.Preview.Length > 200) model.Preview = model.Preview.Substring(0, 200); model.Content = uniqueTitle; } private string ProcessContentHTML(PostViewModel model) { string html; using (WebClient client = new WebClient()) { html = model.Content; } HtmlDocument doc = new HtmlDocument(); doc.LoadHtml(html); try { if (model.Content.Contains( "img" )) { foreach (HtmlNode img in doc.DocumentNode.SelectNodes( "//img" )) { var uploads = Path.Combine(_hostingEnvironment.WebRootPath, "Uploads/BlogImages" ); var base64Img = img.GetAttributeValue( "src" , null ); if (!base64Img.Contains( "Uploads" )) base64Img = base64Img.Split( ';' )[1].Split( ',' )[1].ToString(); if (! string .IsNullOrEmpty(base64Img) && Utility.IsBase64(base64Img)) { var imgSrc = Utility.ConvertBase64ToImage(base64Img, uploads); img.SetAttributeValue( "src" , "/Uploads/BlogImages/" + imgSrc); } } } int i = 0; foreach (HtmlNode hNode in doc.DocumentNode.SelectNodes( "//h1" )) { model.Contents.Add(hNode.InnerText); hNode.SetAttributeValue( "id" , "section_" + i); i++; } using (StringWriter writer = new StringWriter()) { doc.Save(writer); model.Content = writer.ToString(); } } catch { return model.Content; } return model.Content; } private Post ConvertToDTO(PostViewModel model) { Post post = new Post() { ID = model.ID == Guid.Empty ? Guid.NewGuid() : model.ID, ViewCount = model.ID == Guid.Empty ? new Random().Next(50, 100) : model.ViewCount, LikeCount = model.ID == Guid.Empty ? new Random().Next(50, 100) : model.LikeCount, Title = model.Title, Category = model.Category, Series = model.Series, AuthorName = model.AuthorName, Thumbnail = model.Thumbnail, Preview = model.Preview, Content = model.Content, Tags = model.TagsString, Contents = model.Contents, PublishedDate = model.PublishedDate, IsActive = model.IsActive }; return post; } #endregion } } |
EducationManager.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 | using Blog.BAL.Interfaces; using Blog.BAL.Models; using Blog.DAL.Entities; using Microsoft.AspNetCore.Http; using Newtonsoft.Json; using System; using System.Collections.Generic; using System.IO; using Microsoft.AspNetCore.Hosting; namespace Blog.BAL.Implementations { public class EducationManager : IEducationManager { private readonly string contentRootPath = Directory.GetCurrentDirectory(); private readonly IHttpContextAccessor _httpContextAccessor; private readonly IHostingEnvironment _hostingEnvironment; public EducationManager(IHttpContextAccessor httpContextAccessor, IHostingEnvironment hostingEnvironment) { _httpContextAccessor = httpContextAccessor; _hostingEnvironment = hostingEnvironment; } #region private methods private List<Education> ConvertToDTO(List<EducationViewModel> model) { List<Education> educations = new List<Education>(); foreach (var e in model) { Education education = new Education() { School = e.School, Major = e.Major, Location = e.Location, Duration = e.Duration, Items = e.Items }; educations.Add(education); } return educations; } #endregion #region public methods public void EditEducations(List<EducationViewModel> model) { string filePath = $ "{contentRootPath}\\Database\\Educations.json" ; File.WriteAllText(filePath, JsonConvert.SerializeObject(ConvertToDTO(model))); } public List<Education> GetEducations() { string filePath = $ "{contentRootPath}\\Database\\Educations.json" ; List<Education> educations = new List<Education>(); if (File.Exists(filePath)) educations = JsonConvert.DeserializeObject<List<Education>>(System.IO.File.ReadAllText(filePath)); return educations; } public void EditEducations(List<EducationsViewModel> model) { throw new NotImplementedException(); } #endregion } } |
ExperienceManager.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 | using Blog.BAL.Interfaces; using Blog.BAL.Models; using Blog.DAL.Entities; using Microsoft.AspNetCore.Http; using Newtonsoft.Json; using System; using System.Collections.Generic; using System.IO; using Microsoft.AspNetCore.Hosting; namespace Blog.BAL.Implementations { public class EducationManager : IEducationManager { private readonly string contentRootPath = Directory.GetCurrentDirectory(); private readonly IHttpContextAccessor _httpContextAccessor; private readonly IHostingEnvironment _hostingEnvironment; public EducationManager(IHttpContextAccessor httpContextAccessor, IHostingEnvironment hostingEnvironment) { _httpContextAccessor = httpContextAccessor; _hostingEnvironment = hostingEnvironment; } #region private methods private List<Education> ConvertToDTO(List<EducationViewModel> model) { List<Education> educations = new List<Education>(); foreach (var e in model) { Education education = new Education() { School = e.School, Major = e.Major, Location = e.Location, Duration = e.Duration, Items = e.Items }; educations.Add(education); } return educations; } #endregion #region public methods public void EditEducations(List<EducationViewModel> model) { string filePath = $ "{contentRootPath}\\Database\\Educations.json" ; File.WriteAllText(filePath, JsonConvert.SerializeObject(ConvertToDTO(model))); } public List<Education> GetEducations() { string filePath = $ "{contentRootPath}\\Database\\Educations.json" ; List<Education> educations = new List<Education>(); if (File.Exists(filePath)) educations = JsonConvert.DeserializeObject<List<Education>>(System.IO.File.ReadAllText(filePath)); return educations; } public void EditEducations(List<EducationsViewModel> model) { throw new NotImplementedException(); } #endregion } } |
ExperienceManager.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 | using Blog.BAL.Interfaces; using Blog.BAL.Models; using Blog.DAL.Entities; using Microsoft.AspNetCore.Http; using Newtonsoft.Json; using System.Collections.Generic; using System.IO; using Microsoft.AspNetCore.Hosting; namespace Blog.BAL.Implementations { public class ExperienceManager : IExperienceManager { private readonly string contentRootPath = Directory.GetCurrentDirectory(); private readonly IHttpContextAccessor _httpContextAccessor; private readonly IHostingEnvironment _hostingEnvironment; public ExperienceManager(IHttpContextAccessor httpContextAccessor, IHostingEnvironment hostingEnvironment) { _httpContextAccessor = httpContextAccessor; _hostingEnvironment = hostingEnvironment; } #region private methods private List<Experience> ConvertToDTO(List<ExperienceViewModel> model) { List<Experience> experiences = new List<Experience>(); foreach (var exp in model) { Experience experience = new Experience() { Title = exp.Title, Company = exp.Company, Location = exp.Location, Duration = exp.Duration, Items = exp.Items }; experiences.Add(experience); } return experiences; } #endregion #region public methods public void EditExperiences(List<ExperienceViewModel> model) { string filePath = $ "{contentRootPath}\\Database\\Experiences.json" ; File.WriteAllText(filePath, JsonConvert.SerializeObject(ConvertToDTO(model))); } public List<Experience> GetExperiences() { string filePath = $ "{contentRootPath}\\Database\\Experiences.json" ; List<Experience> experiences = new List<Experience>(); if (File.Exists(filePath)) experiences = JsonConvert.DeserializeObject<List<Experience>>(System.IO.File.ReadAllText(filePath)); return experiences; } #endregion } } |
LdObjectManager.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 | using Blog.BAL.Interfaces; using Blog.DAL.Entities; using Microsoft.AspNetCore.Http; using System.Collections.Generic; using System.IO; using Microsoft.AspNetCore.Hosting; namespace Blog.BAL.Implementations { public class LdObjectManager : ILdObjectManager { private readonly string contentRootPath = Directory.GetCurrentDirectory(); private readonly IHttpContextAccessor _httpContextAccessor; private readonly IHostingEnvironment _hostingEnvironment; public LdObjectManager(IHttpContextAccessor httpContextAccessor, IHostingEnvironment hostingEnvironment) { _httpContextAccessor = httpContextAccessor; _hostingEnvironment = hostingEnvironment; } public string GetArticleLdObject(Post post) { ArticleLdJson article = new ArticleLdJson() { mainEntityOfPage = new MainEntityOfPage() { }, headline = post.Title, image = new Image() { url = $ "https://{_httpContextAccessor.HttpContext.Request.Host.Value}/Uploads/BlogImages/" + post.Thumbnail }, datePublished = post.PublishedDate.ToString( "yyyy-MM-dd" ), dateModified = post.UpdatedOn.ToString( "yyyy-MM-dd" ), author = new Author() { name = post.AuthorName }, publisher = new Publisher() { logo = new Logo() { url = $ "https://{_httpContextAccessor.HttpContext.Request.Host.Value}/Uploads/UserImages/blog-icon_269d.jpg" } }, description = post.Preview + "..." }; return article.ToString(); } public string GetOrganizationLdObject() { List<Author> authors = new List<Author>(); authors.Add( new Author() { name = "Hoang (Lucas) Nguyen" }); List< string > sameAs = new List< string >(); OrganizationLdJson org = new OrganizationLdJson() { name = "Lucasology" , logo = $ "https://{_httpContextAccessor.HttpContext.Request.Host.Value}/Uploads/UserImages/blog-icon_269d.jpg" , foundingDate = "2020" , founders = authors, sameAs = sameAs }; return org.ToString(); } public string GetBreadcrumLdObject(List< string > names, List< string > url) { List<BreadcrumElement> elements = new List<BreadcrumElement>(); for ( int i = 0; i < names.Count; i++) { BreadcrumElement e = new BreadcrumElement() { position = i + 1, name = names[i], item = url[i] }; elements.Add(e); } BreadcrumLdJson breadcrum = new BreadcrumLdJson() { itemListElement =elements }; return breadcrum.ToString(); } public string GetCarouselLdObject(List<Post> posts) { List<CarouselElement> elements = new List<CarouselElement>(); int i = 0; foreach (var p in posts) { CarouselElement e = new CarouselElement() { position = i + 1, }; elements.Add(e); i++; } CarouselLdJson carousel = new CarouselLdJson() { itemListElement = elements }; return carousel.ToString(); } } } |
ProjectManager.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 | using Blog.BAL.Interfaces; using Blog.BAL.Models; using Blog.BAL.Helpers; using Blog.DAL.Entities; using Microsoft.AspNetCore.Http; using Newtonsoft.Json; using System; using System.Collections.Generic; using System.IO; using Microsoft.AspNetCore.Hosting; namespace Blog.BAL.Implementations { public class ProjectManager : IProjectManager { private readonly string contentRootPath = Directory.GetCurrentDirectory(); private readonly IHttpContextAccessor _httpContextAccessor; private readonly IHostingEnvironment _hostingEnvironment; public ProjectManager(IHttpContextAccessor httpContextAccessor, IHostingEnvironment hostingEnvironment) { _httpContextAccessor = httpContextAccessor; _hostingEnvironment = hostingEnvironment; } #region private methods private List<Project> ConvertToDTO(List<ProjectViewModel> model) { List<Project> projects = new List<Project>(); foreach (var prj in model) { Project project = new Project() { Name = prj.Name, Description = prj.Description, Image = prj.Image, Links = ConvertToDTO(prj.Links) }; projects.Add(project); } return projects; } private ProjectLinks ConvertToDTO(ProjectLinksViewModel links) { ProjectLinks plList = new ProjectLinks(); foreach (var l in links.Links) { ProjectLink pl = new ProjectLink() { Link = l.Link, LinkName = l.LinkName }; plList.Links.Add(pl); } return plList; } #endregion #region public methods public void EditProjects(List<ProjectViewModel> model) { string filePath = $ "{contentRootPath}\\Database\\Projects.json" ; foreach (var p in model) { if (! string .IsNullOrEmpty(p.Image) && Utility.IsBase64(p.Image)) { var uniqueFileName = Utility.GetUniqueFileName(p.ImageFileName); //Convert Base64 Encoded string to Byte Array. byte [] imageBytes = Convert.FromBase64String(p.Image); //Save the Byte Array as Image File. var uploads = Path.Combine(_hostingEnvironment.WebRootPath, "Uploads/ProjectImages" ); var imgFilePath = Path.Combine(uploads, uniqueFileName); File.WriteAllBytes(imgFilePath, imageBytes); p.Image = uniqueFileName; } if (p.FormFile != null ) { var uniqueFileName = Utility.GetUniqueFileName(p.FormFile.FileName); var uploads = Path.Combine(_hostingEnvironment.WebRootPath, "Uploads/ProjectImages" ); var imgFilePath = Path.Combine(uploads, uniqueFileName); p.FormFile.CopyTo( new FileStream(imgFilePath, FileMode.Create)); p.Image = uniqueFileName; } } File.WriteAllText(filePath, JsonConvert.SerializeObject(ConvertToDTO(model))); } public List<Project> GetProjects() { string filePath = $ "{contentRootPath}\\Database\\Projects.json" ; List<Project> projects = new List<Project>(); if (File.Exists(filePath)) projects = JsonConvert.DeserializeObject<List<Project>>(System.IO.File.ReadAllText(filePath)); return projects; } #endregion } } |
SkillManager.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 | using Blog.BAL.Interfaces; using Blog.BAL.Models; using Blog.DAL.Entities; using Microsoft.AspNetCore.Http; using Newtonsoft.Json; using System; using System.Collections.Generic; using System.IO; using Microsoft.AspNetCore.Hosting; namespace Blog.BAL.Implementations { public class SkillManager : ISkillManager { private readonly string contentRootPath = Directory.GetCurrentDirectory(); private readonly IHttpContextAccessor _httpContextAccessor; private readonly IHostingEnvironment _hostingEnvironment; public SkillManager(IHttpContextAccessor httpContextAccessor, IHostingEnvironment hostingEnvironment) { _httpContextAccessor = httpContextAccessor; _hostingEnvironment = hostingEnvironment; } #region private methods private List<Skill> ConvertToDTO(List<SkillViewModel> model) { List<Skill> skills = new List<Skill>(); foreach (var s in model) { Skill skill = new Skill() { Category = s.Category, Name = s.Name, StartYear = Convert.ToInt32(s.StartYear), Rating = s.Rating }; skills.Add(skill); } return skills; } #endregion #region public methods public void EditSkills(List<SkillViewModel> model) { string filePath = $ "{contentRootPath}\\Database\\Skills.json" ; File.WriteAllText(filePath, JsonConvert.SerializeObject(ConvertToDTO(model))); } public List<Skill> GetSkills() { string filePath = $ "{contentRootPath}\\Database\\Skills.json" ; List<Skill> skills = new List<Skill>(); if (File.Exists(filePath)) skills = JsonConvert.DeserializeObject<List<Skill>>(System.IO.File.ReadAllText(filePath)); return skills; } #endregion } } |
UserManager.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 | using Blog.BAL.Interfaces; using Blog.BAL.Models; using Blog.BAL.Helpers; using Blog.DAL.Entities; using Microsoft.AspNetCore.Http; using Newtonsoft.Json; using System; using System.Collections.Generic; using System.IO; using Microsoft.AspNetCore.Hosting; namespace Blog.BAL.Implementations { public class UserManager : IUserManager { private readonly string contentRootPath = Directory.GetCurrentDirectory(); private readonly IHttpContextAccessor _httpContextAccessor; private readonly IHostingEnvironment _hostingEnvironment; public UserManager(IHttpContextAccessor httpContextAccessor, IHostingEnvironment hostingEnvironment) { _httpContextAccessor = httpContextAccessor; _hostingEnvironment = hostingEnvironment; } #region private methods private User ConvertToDTO(EditAboutMeViewModel model) { User user = new User() { Name = model.Name, Summary = model.Summary, ProfileImage = model.ProfileImage, //Achievements = model.EditAchievement.Achievements, //Skills = ConvertToDTO(model.EditSkill.Skills), //Experiences = ConvertToDTO(model.EditExperience.Experiences), //Educations = ConvertToDTO(model.EditEducation.Educations) }; if ( string .IsNullOrEmpty(model.ProfileImage)) { var owner = GetOwner(); user.ProfileImage = owner.ProfileImage; } return user; } private List<Education> ConvertToDTO(List<EducationViewModel> educations) { List<Education> eduList = new List<Education>(); foreach (var e in educations) { eduList.Add( new Education() { School = e.School, Major = e.Major, Location = e.Location, Duration = e.Duration, Items = e.Items }); } return eduList; } private List<Experience> ConvertToDTO(List<ExperienceViewModel> experiences) { List<Experience> expList = new List<Experience>(); foreach (var e in experiences) { expList.Add( new Experience() { Title = e.Title, Company = e.Company, Location = e.Location, Duration = e.Duration, Items = e.Items }); } return expList; } private List<Skill> ConvertToDTO(List<SkillViewModel> skills) { List<Skill> sList = new List<Skill>(); foreach (var s in skills) { sList.Add( new Skill() { Category = s.Category, Name = s.Name, StartYear = Convert.ToInt32(s.StartYear), Rating = s.Rating }); } return sList; } public bool ValidateUser( string password) { var valid = string .Equals(Utility.EncryptPassword(password, Utility.GetValueByKey( "Salt" )), Utility.GetValueByKey( "HashedPassword" )); return valid; } #endregion #region public methods public void EditAboutMe(EditAboutMeViewModel model) { string filePath = $ "{contentRootPath}\\Database\\Owner.json" ; if (model.FormFile != null ) { var uniqueFileName = Utility.GetUniqueFileName(model.FormFile.FileName); var uploads = Path.Combine(_hostingEnvironment.WebRootPath, "Uploads/UserImages" ); var imgFilePath = Path.Combine(uploads, uniqueFileName); model.FormFile.CopyTo( new FileStream(imgFilePath, FileMode.Create)); model.ProfileImage = uniqueFileName; } File.WriteAllText(filePath, JsonConvert.SerializeObject(ConvertToDTO(model))); } public User GetOwner() { string ownerFilePath = $ "{contentRootPath}\\Database\\Owner.json" ; string experienceFilePath = $ "{contentRootPath}\\Database\\Experiences.json" ; string skillFilePath = $ "{contentRootPath}\\Database\\Skills.json" ; string educationFilePath = $ "{contentRootPath}\\Database\\Educations.json" ; string achievementFilePath = $ "{contentRootPath}\\Database\\Achievements.json" ; string projectFilePath = $ "{contentRootPath}\\Database\\Projects.json" ; User user = null ; if (File.Exists(ownerFilePath)) user = JsonConvert.DeserializeObject<User>(System.IO.File.ReadAllText(ownerFilePath)); if (user != null && File.Exists(experienceFilePath)) user.Experiences = JsonConvert.DeserializeObject<List<Experience>>(System.IO.File.ReadAllText(experienceFilePath)); if (user != null && File.Exists(skillFilePath)) user.Skills = JsonConvert.DeserializeObject<List<Skill>>(System.IO.File.ReadAllText(skillFilePath)); if (user != null && File.Exists(educationFilePath)) user.Educations = JsonConvert.DeserializeObject<List<Education>>(System.IO.File.ReadAllText(educationFilePath)); if (user != null && File.Exists(achievementFilePath)) user.Achievements = JsonConvert.DeserializeObject<List< string >>(System.IO.File.ReadAllText(achievementFilePath)); if (user != null && File.Exists(achievementFilePath)) user.Achievements = JsonConvert.DeserializeObject<List< string >>(System.IO.File.ReadAllText(achievementFilePath)); if (user != null && File.Exists(projectFilePath)) user.Projects = JsonConvert.DeserializeObject<List<Project>>(System.IO.File.ReadAllText(projectFilePath)); return user; } public bool Login(LoginViewModel model) { bool valid = false ; if (ValidateUser(model.Password)) valid = true ; return valid; } #endregion } } |
3. In Interfaces folder, create below classes
IAchievementManager.cs
1 2 3 4 5 6 7 8 9 10 11 | using System.Collections.Generic; namespace Blog.BAL.Interfaces { public interface IAchievementManager { void EditAchievements(List< string > model); List< string > GetAchievements(); } } |
IBlogManager.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | using Blog.BAL.Models; using Blog.DAL.Entities; using System.Collections.Generic; namespace Blog.BAL.Interfaces { public interface IBlogManager { void Create(PostViewModel model); List<Post> GetPosts( int ? take); Post GetPostByUrl( string url); string GetContentByUrl( string url); void Edit(PostViewModel model); Post GetPostByUrlForArchival( string url); List<Post> GetAllPosts( int ? take); } } |
IEducationManager.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 | using Blog.BAL.Models; using Blog.DAL.Entities; using System.Collections.Generic; namespace Blog.BAL.Interfaces { public interface IEducationManager { void EditEducations(List<EducationViewModel> model); List<Education> GetEducations(); } } |
IExperienceManager.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 | using Blog.BAL.Models; using Blog.DAL.Entities; using System.Collections.Generic; namespace Blog.BAL.Interfaces { public interface IExperienceManager { void EditExperiences(List<ExperienceViewModel> model); List<Experience> GetExperiences(); } } |
ILdObjectManager.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | using Blog.DAL.Entities; using System.Collections.Generic; namespace Blog.BAL.Interfaces { public interface ILdObjectManager { string GetArticleLdObject(Post post); string GetOrganizationLdObject(); string GetBreadcrumLdObject(List< string > names, List< string > urls); string GetCarouselLdObject(List<Post> posts); } } |
IProjectManager.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 | using Blog.BAL.Models; using Blog.DAL.Entities; using System.Collections.Generic; namespace Blog.BAL.Interfaces { public interface IProjectManager { void EditProjects(List<ProjectViewModel> model); List<Project> GetProjects(); } } |
ISkillManager.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 | using Blog.BAL.Models; using Blog.DAL.Entities; using System.Collections.Generic; namespace Blog.BAL.Interfaces { public interface ISkillManager { void EditSkills(List<SkillViewModel> model); List<Skill> GetSkills(); } } |
IUserManager.cs
1 2 3 4 5 6 7 8 9 10 11 12 | using Blog.BAL.Models; using Blog.DAL.Entities; namespace Blog.BAL.Interfaces { public interface IUserManager { void EditAboutMe(EditAboutMeViewModel user); User GetOwner(); bool Login(LoginViewModel model); } } |
3. In Models folder, create below classes
AchievementViewModel.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 | using System.Collections.Generic; using System.ComponentModel.DataAnnotations; namespace Blog.BAL.Models { public class EditAchievementViewModel { public EditAchievementViewModel() { Achievements = new List< string >(); } [Required] public string Achievement { get ; set ; } public List< string > Achievements { get ; set ; } } public class AchievementsViewModel { public List< string > Achievements { get ; set ; } } } |
BlogViewModel.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | using Blog.BAL.Helpers; using System.Collections.Generic; namespace Blog.BAL.Models { public class BlogViewModel { public List< string > Categories { get ; set ; } public PaginatedList<PostViewModel> PagedBlog { get ; set ; } public List<PostViewModel> FavoritePosts { get ; set ; } public List<PostViewModel> PopularPosts { get ; set ; } public UserViewModel AboutMe { get ; set ; } } } |
EducationViewModel.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 | using System; using System.Collections.Generic; using System.ComponentModel.DataAnnotations; namespace Blog.BAL.Models { public class EducationViewModel { public Guid ID { get ; set ; } public string School { get ; set ; } public string Major { get ; set ; } public string Location { get ; set ; } public string Duration { get ; set ; } public string Items { get ; set ; } } public class EducationsViewModel { public List<EducationViewModel> Educations { get ; set ; } } public class EditEducationViewModel { public EditEducationViewModel() { Educations = new List<EducationViewModel>(); } [Required] public string School { get ; set ; } [Required] public string Major { get ; set ; } [Required] public string Location { get ; set ; } [Required] public string Duration { get ; set ; } [Required] public string Items { get ; set ; } public List<EducationViewModel> Educations { get ; set ; } } } |
ExperienceViewModel.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 | using System; using System.Collections.Generic; using System.ComponentModel.DataAnnotations; namespace Blog.BAL.Models { public class EducationViewModel { public Guid ID { get ; set ; } public string School { get ; set ; } public string Major { get ; set ; } public string Location { get ; set ; } public string Duration { get ; set ; } public string Items { get ; set ; } } public class EducationsViewModel { public List<EducationViewModel> Educations { get ; set ; } } public class EditEducationViewModel { public EditEducationViewModel() { Educations = new List<EducationViewModel>(); } [Required] public string School { get ; set ; } [Required] public string Major { get ; set ; } [Required] public string Location { get ; set ; } [Required] public string Duration { get ; set ; } [Required] public string Items { get ; set ; } public List<EducationViewModel> Educations { get ; set ; } } } |
LoginViewModel.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 | using System.ComponentModel.DataAnnotations; namespace Blog.BAL.Models { public class LoginViewModel { [Required] public string Password { get ; set ; } public bool RememberMe { get ; set ; } } } |
PostViewModel.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 | using Microsoft.AspNetCore.Http; using System; using System.Collections.Generic; using System.ComponentModel.DataAnnotations; namespace Blog.BAL.Models { public class PostViewModel { public Guid ID { get ; set ; } [Required] public string Title { get ; set ; } public string Series { get ; set ; } public int ViewCount { get ; set ; } public int LikeCount { get ; set ; } [Required] public string Category { get ; set ; } [Required] public string AuthorName { get ; set ; } = "Lucas" ; [Display(Name = "Thumbnail" )] public IFormFile FormFile { get ; set ; } public string Thumbnail { get ; set ; } [Required] public string Content { get ; set ; } public string Preview { get ; set ; } [Required] public string TagsString { get ; set ; } public List< string > Tags { get ; set ; } [Required] public DateTime PublishedDate { get ; set ; } = DateTime.Now; public List< string > Contents { get ; set ; } = new List< string >(); public List<PostViewModel> SeriesPosts { get ; set ; } = new List<PostViewModel>(); public bool IsActive { get ; set ; } } } |
ProjectViewModel.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 | using Microsoft.AspNetCore.Http; using System; using System.Collections.Generic; using System.ComponentModel.DataAnnotations; namespace Blog.BAL.Models { public class ProjectViewModel { public ProjectViewModel() { Links = new ProjectLinksViewModel(); } public Guid ID { get ; set ; } [Display(Name = "Profile Image" )] public IFormFile FormFile { get ; set ; } public string Image { get ; set ; } public string ImageFileName { get ; set ; } [Required] public string Name { get ; set ; } [Required] public string Description { get ; set ; } public ProjectLinksViewModel Links { get ; set ; } } public class ProjectsViewModel { public List<ProjectViewModel> Projects { get ; set ; } } public class ProjectLinkViewModel { [Required] public string Link { get ; set ; } [Required] public string LinkName { get ; set ; } } public class ProjectLinksViewModel { public ProjectLinksViewModel() { Links = new List<ProjectLinkViewModel>(); } public List<ProjectLinkViewModel> Links { get ; set ; } } public class EditPortfolioViewModel { public EditPortfolioViewModel() { Links = new ProjectLinksViewModel(); Projects = new List<ProjectViewModel>(); } [Display(Name = "Project Image" )] public IFormFile FormFile { get ; set ; } public string Image { get ; set ; } public string ImageFileName { get ; set ; } [Required] public string Name { get ; set ; } [Required] public string Description { get ; set ; } public ProjectLinksViewModel Links { get ; set ; } public List<ProjectViewModel> Projects { get ; set ; } } } |
SkillViewModel.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 | using System; using System.Collections.Generic; using System.ComponentModel.DataAnnotations; namespace Blog.BAL.Models { public class SkillViewModel { public Guid ID { get ; set ; } [Required] public string Category { get ; set ; } [Required] public string Name { get ; set ; } [Required] public double ? StartYear { get ; set ; } public double ? Rating { get ; set ; } } public class SkillsViewModel { public List<SkillViewModel> Skills { get ; set ; } } public class EditSkillViewModel { public EditSkillViewModel() { Skills = new List<SkillViewModel>(); } [Required] public string Category { get ; set ; } [Required] public string Name { get ; set ; } [Required] public double ? StartYear { get ; set ; } public double ? Rating { get ; set ; } public List<SkillViewModel> Skills { get ; set ; } } } |
UserViewModel.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 | using Microsoft.AspNetCore.Http; using System; using System.Collections.Generic; using System.ComponentModel.DataAnnotations; namespace Blog.BAL.Models { public class UserViewModel { public Guid ID { get ; set ; } public string ProfileImage { get ; set ; } public string Name { get ; set ; } public string Summary { get ; set ; } public List< string > Achievements { get ; set ; } public List<SkillViewModel> Skills { get ; set ; } public List<ExperienceViewModel> Experiences { get ; set ; } public List<EducationViewModel> Educations { get ; set ; } public List<ProjectViewModel> Projects { get ; set ; } public List<PostViewModel> Posts { get ; set ; } } public class EditUserViewModel { public EditUserViewModel() { EditAchievement = new EditAchievementViewModel(); EditSkill = new EditSkillViewModel(); EditExperience = new EditExperienceViewModel(); EditEducation = new EditEducationViewModel(); EditPortfolio = new EditPortfolioViewModel(); } public Guid ID { get ; set ; } public EditAboutMeViewModel EditAboutMe { get ; set ; } public EditAchievementViewModel EditAchievement { get ; set ; } public EditSkillViewModel EditSkill { get ; set ; } public EditExperienceViewModel EditExperience { get ; set ; } public EditEducationViewModel EditEducation { get ; set ; } public EditPortfolioViewModel EditPortfolio { get ; set ; } } public class EditAboutMeViewModel { [Display(Name = "Profile Image" )] public IFormFile FormFile { get ; set ; } public string ProfileImage { get ; set ; } [Required] public string Name { get ; set ; } [Required] public string Summary { get ; set ; } } } |
Step 3: Build Blog.Web project
Install below package from Manage NuGet Packages:
- AutoMapper.Extensions.Microsoft.DependencyInjection (8.1.0)
- Microsoft.AspNetCore.All (2.0.9)
- Microsoft.VisualStudio.Web.CodeGeneration.Design (2.0.4)
Install below client library by right click on project >> Add >> Client Side Library:
- bootstrap 4.3.1
- font-awesome
- summernote
- SyntaxHighlighter
This project has below structure:
Program.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | using Microsoft.AspNetCore; using Microsoft.AspNetCore.Hosting; namespace Blog.Web { public class Program { public static void Main( string [] args) { BuildWebHost(args).Run(); } public static IWebHost BuildWebHost( string [] args) => WebHost.CreateDefaultBuilder(args) .UseStartup<Startup>() .Build(); } } |
Startup.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 | using System; using AutoMapper; using Blog.BAL.Helpers; using Blog.BAL.Implementations; using Blog.BAL.Interfaces; using Blog.Web.Infrastructures; using Microsoft.AspNetCore.Authentication.Cookies; using Microsoft.AspNetCore.Authorization; using Microsoft.AspNetCore.Builder; using Microsoft.AspNetCore.Hosting; using Microsoft.AspNetCore.Http; using Microsoft.AspNetCore.Identity; using Microsoft.AspNetCore.Mvc.Razor; using Microsoft.Extensions.Caching.Memory; using Microsoft.Extensions.Configuration; using Microsoft.Extensions.DependencyInjection; namespace Blog.Web { public class Startup { public Startup(IConfiguration configuration) { Configuration = configuration; } public IConfiguration Configuration { get ; } // This method gets called by the runtime. Use this method to add services to the container. public void ConfigureServices(IServiceCollection services) { //AutoMapper services.AddAutoMapper( typeof (Startup)); //Dependency Injection services.AddScoped<IUserManager, UserManager>(); services.AddScoped<ISkillManager, SkillManager>(); services.AddScoped<IAchievementManager, AchievementManager>(); services.AddScoped<IEducationManager, EducationManager>(); services.AddScoped<IExperienceManager, ExperienceManager>(); services.AddScoped<IProjectManager, ProjectManager>(); services.AddScoped<IBlogManager, BlogManager>(); services.AddScoped<ILdObjectManager, LdObjectManager>(); //HttpContextAccessor services.AddHttpContextAccessor(); services.Configure<SecurityStampValidatorOptions>(options => { options.ValidationInterval = TimeSpan.FromDays(365); }); services.AddAuthentication(CookieAuthenticationDefaults.AuthenticationScheme) .AddCookie(config => { config.Cookie.Name = "__MyBlogCookie__" ; config.LoginPath = "/Home/Login" ; config.SlidingExpiration = true ; config.Cookie.SecurePolicy = CookieSecurePolicy.SameAsRequest; config.Cookie.Expiration = TimeSpan.FromSeconds(10); config.ExpireTimeSpan = TimeSpan.FromSeconds(10); }); services.AddAuthorization(); //AMP services.Configure<RazorViewEngineOptions>(options => { options.ViewLocationExpanders.Add( new AmpViewLocationExpander()); }); services.AddMvc(options => { var policy = new AuthorizationPolicyBuilder() .RequireAuthenticatedUser() .Build(); }); } // This method gets called by the runtime. Use this method to configure the HTTP request pipeline. public void Configure(IApplicationBuilder app, IHostingEnvironment env) { if (env.IsDevelopment()) { app.UseBrowserLink(); app.UseDeveloperExceptionPage(); } else { app.UseExceptionHandler( "/Home/Error" ); } app.UseStaticFiles(); app.UseAuthentication(); MyAppContext.Configure(app.ApplicationServices.GetRequiredService<IHttpContextAccessor>(), app.ApplicationServices.GetRequiredService<IMemoryCache>()); MyAppContext.SetupRefreshJob(); app.UseStatusCodePagesWithReExecute( "/Error/{0}" ); app.UseMvc(routes => { routes.MapRoute( name: "default" , template: "{controller=Home}/{action=Index}/{id?}" ); }); } } } |
appsettings.json
1 2 3 4 5 6 7 8 9 10 11 12 13 | { "Logging" : { "IncludeScopes" : false , "LogLevel" : { "Default" : "Warning" } }, "AllowedHosts" : "*" , "StartYear" : "2015" , "HashedPassword" : "Your Password" , "Salt" : "Your Salt" , "Version" : "1.0.0" } |
1. In Controllers folder, create below controllers
AboutMeController.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 369 370 371 372 373 374 375 376 377 378 379 380 381 382 383 384 385 386 387 388 389 390 391 392 393 394 395 396 397 398 399 400 401 402 403 404 405 406 407 408 409 410 411 412 413 414 415 416 417 418 419 420 421 422 423 424 425 426 427 428 429 430 431 432 433 434 435 436 437 438 439 440 441 442 443 444 445 446 447 448 449 450 451 452 453 454 455 456 457 458 459 460 461 462 463 464 465 466 467 468 469 470 471 472 473 474 475 476 477 478 479 480 481 482 483 484 485 486 487 488 489 490 491 492 493 494 495 496 497 498 499 500 501 502 503 504 505 506 507 508 509 510 511 512 513 514 515 516 517 518 519 520 521 522 523 524 525 526 527 528 529 530 531 532 533 534 535 536 537 538 539 540 541 542 543 544 545 546 547 548 549 550 551 552 553 554 555 556 557 558 559 560 561 562 563 564 565 566 567 568 569 570 571 572 573 574 575 576 577 578 579 580 581 582 583 584 585 586 587 588 589 590 591 592 593 594 595 596 597 598 599 600 601 602 603 604 605 606 607 608 609 610 611 612 613 614 615 616 617 618 619 620 621 622 623 624 625 626 627 628 629 630 631 632 633 634 635 636 637 638 639 640 641 642 643 644 | using AutoMapper; using Blog.BAL.Interfaces; using Blog.BAL.Models; using Blog.BAL.Helpers; using Blog.DAL.Entities; using Microsoft.AspNetCore.Http; using Microsoft.AspNetCore.Mvc; using Microsoft.Extensions.Configuration; using System; using System.Collections.Generic; using Microsoft.AspNetCore.Authorization; using System.Security.Claims; using Microsoft.AspNetCore.Authentication; using Microsoft.AspNetCore.Authentication.Cookies; namespace Blog.Web.Controllers { public class AboutMeController : Controller { private readonly IConfiguration _config; private readonly IUserManager _userManager; private readonly ISkillManager _skillManager; private readonly IExperienceManager _experienceManager; private readonly IEducationManager _educationManager; private readonly IAchievementManager _achievementManager; private readonly IProjectManager _projectManager; private readonly IBlogManager _blogManager; private readonly ILdObjectManager _ldObjectManager; private readonly IMapper _mapper; private readonly IHttpContextAccessor _httpContextAccessor; public AboutMeController(IConfiguration config, IUserManager userManager, ISkillManager skillManager, IExperienceManager experienceManager, IEducationManager educationManager, IAchievementManager achievementManager, IProjectManager projectManager, IBlogManager blogManager, ILdObjectManager ldObjectManager, IMapper mapper, IHttpContextAccessor httpContextAccessor) { _config = config; _userManager = userManager; _skillManager = skillManager; _experienceManager = experienceManager; _educationManager = educationManager; _achievementManager = achievementManager; _projectManager = projectManager; _blogManager = blogManager; _ldObjectManager = ldObjectManager; _mapper = mapper; _httpContextAccessor = httpContextAccessor; } // GET: AboutMeController public ActionResult Index() { User owner = _userManager.GetOwner(); var model = _mapper.Map<UserViewModel>(owner); model.Posts = _mapper.Map<List<PostViewModel>>(_blogManager.GetPosts(3)); ViewBag.LdJsonObject = _ldObjectManager.GetOrganizationLdObject(); ViewBag.ArticleTitle = " About Me" ; ViewBag.Thumbnail = "/images/LucaSharp-big.jpg" ; ViewBag.TwitterThumbnail = $ "http://{_httpContextAccessor.HttpContext.Request.Host.Value}/images/LucaSharp-big.jpg" ; ViewBag.Preview = "Experienced Software Engineer with a demonstrated history of working in technology industry. Skilled in .NET Framework, MS SQL Server, C#, AngularJS, and Web API/Services. Strong engineering professional with a Bachelor of Science (B.S.) focused in Computer Information Systems." ; return View(model); } // GET: AboutMeController/Create [Authorize] public ActionResult EditProfile() { EditUserViewModel model = new EditUserViewModel(); var owner = _userManager.GetOwner(); if (owner != null ) model.EditAboutMe = _mapper.Map<EditAboutMeViewModel>(owner); var skills = _skillManager.GetSkills(); if (skills.Count > 0) model.EditSkill.Skills = _mapper.Map<List<SkillViewModel>>(skills); var experiences = _experienceManager.GetExperiences(); if (experiences.Count > 0) model.EditExperience.Experiences = _mapper.Map<List<ExperienceViewModel>>(experiences); var educations = _educationManager.GetEducations(); if (educations.Count > 0) model.EditEducation.Educations = _mapper.Map<List<EducationViewModel>>(educations); var achievements = _achievementManager.GetAchievements(); if (achievements.Count > 0) model.EditAchievement.Achievements = achievements; var projects = _projectManager.GetProjects(); if (projects.Count > 0) model.EditPortfolio.Projects = _mapper.Map<List<ProjectViewModel>>(projects); return View(model); } private List<ProjectLinkViewModel> ConvertToVM(List<ProjectLink> links) { List<ProjectLinkViewModel> plList = new List<ProjectLinkViewModel>(); foreach (var pl in links) { ProjectLinkViewModel plVM = new ProjectLinkViewModel() { Link = pl.Link, LinkName = pl.LinkName }; plList.Add(plVM); } return plList; } // POST: AboutMeController/Create [HttpPost] //[ValidateAntiForgeryToken] public ActionResult SaveAboutMe(EditAboutMeViewModel model) { try { if (ModelState.IsValid) { _userManager.EditAboutMe(model); } return PartialView( "_EditAboutMe" , model); } catch { return PartialView( "_EditAboutMe" , model); } } [HttpPost] //[ValidateAntiForgeryToken] public ActionResult SaveSkills(SkillsViewModel model) { try { if (ModelState.IsValid) { _skillManager.EditSkills(model.Skills); } EditSkillViewModel m = new EditSkillViewModel(); m.Skills = model.Skills; return PartialView( "_EditSkill" , m); } catch { return PartialView( "_EditSkill" , model); } } [HttpPost] //[ValidateAntiForgeryToken] public ActionResult AddSkill(EditSkillViewModel model) { try { if (ModelState.IsValid) { SkillViewModel m = new SkillViewModel() { Category = model.Category, Name = model.Name, StartYear = Convert.ToInt32(model.StartYear), Rating = Utility.CalculateSkillRating(model.StartYear) }; model.Skills.Add(m); } return PartialView( "_EditSkill" , model); } catch { ModelState.AddModelError( "" , "Something Wrong. Please Try Again!" ); return PartialView( "_EditSkill" , model); } } [HttpPost] //[ValidateAntiForgeryToken] public ActionResult RemoveSkill(SkillsViewModel model, int id) { try { ModelState.Clear(); //need to rebuild model to pass to view model.Skills.RemoveAt(id); EditSkillViewModel m = new EditSkillViewModel(); m.Skills.AddRange(model.Skills); return PartialView( "_EditSkill" , m); } catch { ModelState.AddModelError( "" , "Something Wrong. Please Try Again!" ); return PartialView( "_EditSkill" , model); } } [HttpPost] //[ValidateAntiForgeryToken] public ActionResult UpdateSkill(SkillsViewModel model, int id) { try { ModelState.Clear(); //need to rebuild model to pass to view model.Skills[id].Rating = Utility.CalculateSkillRating(model.Skills[id].StartYear); EditSkillViewModel m = new EditSkillViewModel(); m.Skills.AddRange(model.Skills); return PartialView( "_EditSkill" , m); } catch { ModelState.AddModelError( "" , "Something Wrong. Please Try Again!" ); return PartialView( "_EditSkill" , model); } } [HttpPost] //[ValidateAntiForgeryToken] public ActionResult SaveExperiences(ExperiencesViewModel model) { try { if (ModelState.IsValid) { _experienceManager.EditExperiences(model.Experiences); } EditExperienceViewModel m = new EditExperienceViewModel(); m.Experiences = model.Experiences; return PartialView( "_EditExperience" , m); } catch { return PartialView( "_EditExperience" , model); } } [HttpPost] //[ValidateAntiForgeryToken] public ActionResult AddExp(EditExperienceViewModel model) { try { if (ModelState.IsValid) { ExperienceViewModel m = new ExperienceViewModel() { Title = model.Title, Company = model.Company, Location = model.Location, Duration = model.Duration, Items = model.Items }; model.Experiences.Add(m); } return PartialView( "_EditExperience" , model); } catch { ModelState.AddModelError( "" , "Something Wrong. Please Try Again!" ); return PartialView( "_EditExperience" , model); } } [HttpPost] //[ValidateAntiForgeryToken] public ActionResult RemoveExp(ExperiencesViewModel model, int id) { try { ModelState.Clear(); //need to rebuild model to pass to view model.Experiences.RemoveAt(id); EditExperienceViewModel m = new EditExperienceViewModel(); m.Experiences.AddRange(model.Experiences); return PartialView( "_EditExperience" , m); } catch { ModelState.AddModelError( "" , "Something Wrong. Please Try Again!" ); return PartialView( "_EditExperience" , model); } } [HttpPost] //[ValidateAntiForgeryToken] public ActionResult UpdateExp(ExperiencesViewModel model, int id) { try { ModelState.Clear(); //need to rebuild model to pass to view EditExperienceViewModel m = new EditExperienceViewModel(); m.Experiences.AddRange(model.Experiences); return PartialView( "_EditExperience" , m); } catch { ModelState.AddModelError( "" , "Something Wrong. Please Try Again!" ); return PartialView( "_EditExperience" , model); } } [HttpPost] //[ValidateAntiForgeryToken] public ActionResult SaveEducations(EducationsViewModel model) { try { if (ModelState.IsValid) { _educationManager.EditEducations(model.Educations); } EditEducationViewModel m = new EditEducationViewModel(); m.Educations = model.Educations; return PartialView( "_EditEducation" , m); } catch { return PartialView( "_EditEducation" , model); } } [HttpPost] //[ValidateAntiForgeryToken] public ActionResult AddEdu(EditEducationViewModel model) { try { if (ModelState.IsValid) { EducationViewModel m = new EducationViewModel() { School = model.School, Major = model.Major, Location = model.Location, Duration = model.Duration, Items = model.Items }; model.Educations.Add(m); } return PartialView( "_EditEducation" , model); } catch { ModelState.AddModelError( "" , "Something Wrong. Please Try Again!" ); return PartialView( "_EditEducation" , model); } } [HttpPost] //[ValidateAntiForgeryToken] public ActionResult RemoveEdu(EducationsViewModel model, int id) { try { ModelState.Clear(); //need to rebuild model to pass to view model.Educations.RemoveAt(id); EditEducationViewModel m = new EditEducationViewModel(); m.Educations.AddRange(model.Educations); return PartialView( "_EditEducation" , m); } catch { ModelState.AddModelError( "" , "Something Wrong. Please Try Again!" ); return PartialView( "_EditEducation" , model); } } [HttpPost] //[ValidateAntiForgeryToken] public ActionResult UpdateEdu(EducationsViewModel model, int id) { try { ModelState.Clear(); //need to rebuild model to pass to view EditEducationViewModel m = new EditEducationViewModel(); m.Educations.AddRange(model.Educations); return PartialView( "_EditEducation" , m); } catch { ModelState.AddModelError( "" , "Something Wrong. Please Try Again!" ); return PartialView( "_EditEducation" , model); } } [HttpPost] //[ValidateAntiForgeryToken] public ActionResult SaveAchievements(AchievementsViewModel model) { try { if (ModelState.IsValid) { _achievementManager.EditAchievements(model.Achievements); } EditAchievementViewModel m = new EditAchievementViewModel(); m.Achievements = model.Achievements; return PartialView( "_EditAchievement" , m); } catch { return PartialView( "_EditAchievement" , model); } } [HttpPost] //[ValidateAntiForgeryToken] public ActionResult AddAchievement(EditAchievementViewModel model) { try { if (ModelState.IsValid) { model.Achievements.Add(model.Achievement); } return PartialView( "_EditAchievement" , model); } catch { ModelState.AddModelError( "" , "Something Wrong. Please Try Again!" ); return PartialView( "_EditAchievement" , model); } } [HttpPost] //[ValidateAntiForgeryToken] public ActionResult RemoveAchievement(AchievementsViewModel model, int id) { try { ModelState.Clear(); //need to rebuild model to pass to view model.Achievements.RemoveAt(id); EditAchievementViewModel m = new EditAchievementViewModel(); m.Achievements.AddRange(model.Achievements); return PartialView( "_EditAchievement" , m); } catch { ModelState.AddModelError( "" , "Something Wrong. Please Try Again!" ); return PartialView( "_EditAchievement" , model); } } [HttpPost] //[ValidateAntiForgeryToken] public ActionResult UpdateAchievement(AchievementsViewModel model, int id) { try { ModelState.Clear(); //need to rebuild model to pass to view EditAchievementViewModel m = new EditAchievementViewModel(); m.Achievements.AddRange(model.Achievements); return PartialView( "_EditAchievement" , m); } catch { ModelState.AddModelError( "" , "Something Wrong. Please Try Again!" ); return PartialView( "_EditAchievement" , model); } } [HttpPost] //[ValidateAntiForgeryToken] public ActionResult AddProjectLink(ProjectLinksViewModel model) { try { if (model.Links == null ) model.Links = new List<ProjectLinkViewModel>(); if (model.Links != null && ModelState.IsValid) { model.Links.Add( new ProjectLinkViewModel()); } return PartialView( "_EditProjectLinks" , model); } catch { return PartialView( "_EditProjectLinks" , model); } } [HttpPost] //[ValidateAntiForgeryToken] public ActionResult RemoveProjectLink(ProjectLinksViewModel model, int id) { try { ModelState.Clear(); //need to rebuild model to pass to view model.Links.RemoveAt(id); return PartialView( "_EditProjectLinks" , model); } catch { ModelState.AddModelError( "" , "Something Wrong. Please Try Again!" ); return PartialView( "_EditProjectLinks" , model); } } public ActionResult Login() { return PartialView( "_Login" ); } [HttpPost] //[ValidateAntiForgeryToken] public ActionResult AddProject(EditPortfolioViewModel model) { try { if (ModelState.IsValid) { ProjectViewModel m = new ProjectViewModel() { Name = model.Name, Description = model.Description, Image = model.FormFile != null ? Utility.ConvertToBase64Image(model.FormFile) : "" , ImageFileName = model.FormFile != null ? model.FormFile.FileName : "" , Links = model.Links }; model.Links = new ProjectLinksViewModel(); model.Projects.Add(m); } return PartialView( "_EditPortfolio" , model); } catch { ModelState.AddModelError( "" , "Something Wrong. Please Try Again!" ); return PartialView( "_EditPortfolio" , model); } } [HttpPost] //[ValidateAntiForgeryToken] public ActionResult UpdateProject(ProjectsViewModel model, int prjIndex) { try { ModelState.Clear(); //need to rebuild model to pass to view model.Projects[prjIndex].Image = model.Projects[prjIndex].FormFile != null ? Utility.ConvertToBase64Image(model.Projects[prjIndex].FormFile) : model.Projects[prjIndex].Image; model.Projects[prjIndex].ImageFileName = model.Projects[prjIndex].FormFile != null ? model.Projects[prjIndex].FormFile.FileName : model.Projects[prjIndex].ImageFileName; EditPortfolioViewModel m = new EditPortfolioViewModel(); m.Projects.AddRange(model.Projects); return PartialView( "_EditPortfolio" , m); } catch { ModelState.AddModelError( "" , "Something Wrong. Please Try Again!" ); return PartialView( "_EditPortfolio" , model); } } [HttpPost] //[ValidateAntiForgeryToken] public ActionResult RemoveProject(ProjectsViewModel model, int prjIndex) { try { ModelState.Clear(); //need to rebuild model to pass to view model.Projects.RemoveAt(prjIndex); EditPortfolioViewModel m = new EditPortfolioViewModel(); m.Projects.AddRange(model.Projects); return PartialView( "_EditPortfolio" , m); } catch { ModelState.AddModelError( "" , "Something Wrong. Please Try Again!" ); return PartialView( "_EditPortfolio" , model); } } [HttpPost] //[ValidateAntiForgeryToken] public ActionResult SavePortfolios(ProjectsViewModel model) { try { if (ModelState.IsValid) { _projectManager.EditProjects(model.Projects); } EditPortfolioViewModel m = new EditPortfolioViewModel(); m.Projects = model.Projects; return PartialView( "_EditPortfolio" , m); } catch (Exception ex) { return PartialView( "_EditPortfolio" , model); } } [HttpPost] //[ValidateAntiForgeryToken] public ActionResult Login(LoginViewModel model) { try { bool valid = false ; if (ModelState.IsValid) valid = _userManager.Login(model); if (valid) { MySession.Set(MyAppContext.Current, "__MyBlogCookie__" , Guid.NewGuid().ToString(), model.RememberMe ? DateTime.UtcNow.AddDays(365) : DateTime.UtcNow.AddSeconds(5)); var userClaims = new List<Claim>() { new Claim(ClaimTypes.Name, "Hoang" ) }; var identity = new ClaimsIdentity(userClaims, CookieAuthenticationDefaults.AuthenticationScheme); HttpContext.SignInAsync( new ClaimsPrincipal(identity), new AuthenticationProperties { IsPersistent = model.RememberMe, ExpiresUtc = model.RememberMe ? DateTime.UtcNow.AddDays(365) : DateTime.UtcNow.AddSeconds(5) }); } else ModelState.AddModelError( "" , "Incorrect Password!" ); return PartialView( "_Login" , model); } catch { ModelState.AddModelError( "" , "Something Wrong. Please Try Again!" ); return PartialView( "_Login" , model); } } public ActionResult LogOut() { HttpContext.SignOutAsync( "__MyBlogCookie__" ); MySession.Remove(MyAppContext.Current, "__MyBlogCookie__" ); return RedirectToAction( "Index" , "Home" ); } } } |
BlogController.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 | using System; using System.Collections.Generic; using System.Linq; using System.Threading.Tasks; using AutoMapper; using Blog.BAL.Helpers; using Blog.BAL.Interfaces; using Blog.BAL.Models; using Blog.DAL.Entities; using Microsoft.AspNetCore.Authorization; using Microsoft.AspNetCore.Http; using Microsoft.AspNetCore.Mvc; namespace Blog.Web.Controllers { public class BlogController : Controller { private readonly IBlogManager _blogManager; private readonly IUserManager _userManager; private readonly ILdObjectManager _ldObjectManager; private readonly IHttpContextAccessor _httpContextAccessor; private readonly IMapper _mapper; public BlogController(IBlogManager blogerManager, IMapper mapper, IUserManager userManager, ILdObjectManager ldObjectManager, IHttpContextAccessor httpContextAccessor) { _blogManager = blogerManager; _userManager = userManager; _mapper = mapper; _ldObjectManager = ldObjectManager; _httpContextAccessor = httpContextAccessor; } // GET: BlogController public async Task<IActionResult> Index( string currentFilter, string searchString, string tagFilter, string catFilter, int ? pageNumber) { var model = new BlogViewModel(); if (searchString != null ) { pageNumber = 1; } else { searchString = currentFilter; } ViewData[ "CurrentFilter" ] = searchString; var posts = _blogManager.GetPosts( null ).OrderByDescending(p => p.PublishedDate).ToList(); var favPosts = posts.OrderByDescending(p => p.LikeCount).Take(5).ToList(); var popularPosts = posts.OrderByDescending(p => p.ViewCount).Take(5).ToList(); var aboutMe = _userManager.GetOwner(); model.Categories = posts.Select(p => p.Category).Distinct().ToList(); model.Categories.Add( "All" ); if (! string .IsNullOrEmpty(searchString)) { posts = posts.Where(p => p.Title.ToUpper().Contains(searchString.ToUpper()) || p.Category.ToUpper().Contains(searchString.ToUpper()) || p.AuthorName.ToUpper().Contains(searchString.ToUpper()) || p.Series.ToUpper().Contains(searchString.ToUpper()) || p.Preview.ToUpper().Contains(searchString.ToUpper()) || p.Tags.ToUpper().Contains(searchString.ToUpper())).ToList(); } if (! string .IsNullOrEmpty(tagFilter)) { ViewData[ "tagFilter" ] = tagFilter; posts = posts.Where(p => p.Tags.Contains(tagFilter)).ToList(); } ViewBag.LdJsonObject = _ldObjectManager.GetCarouselLdObject(posts); if (! string .IsNullOrEmpty(catFilter)) { var catUrl = $ "https://{_httpContextAccessor.HttpContext.Request.Host.Value}/?catFilter={catFilter}" ; ViewBag.LdJsonObject = _ldObjectManager.GetBreadcrumLdObject( new List< string > { "Blog" , catFilter }, new List< string > { url, catUrl }); ViewData[ "catFilter" ] = catFilter; posts = posts.Where(p => p.Category.ToUpper() == catFilter.ToUpper()).ToList(); } int pageSize = 10; model.PagedBlog = PaginatedList<PostViewModel>.Create(_mapper.Map<List<PostViewModel>>(posts), pageNumber ?? 1, pageSize); model.FavoritePosts = _mapper.Map<List<PostViewModel>>(favPosts); model.PopularPosts = _mapper.Map<List<PostViewModel>>(popularPosts); model.AboutMe = _mapper.Map<UserViewModel>(aboutMe); return View(await Task.FromResult(model)); } [Authorize] public async Task<IActionResult> Dashboard( string sortOrder, string currentFilter, string searchString, int ? pageNumber) { ViewData[ "TitleSortParm" ] = sortOrder == "title" ? "title_desc" : "title" ; ViewData[ "CategorySortParm" ] = sortOrder == "category" ? "category_desc" : "category" ; ViewData[ "SeriesSortParm" ] = sortOrder == "series" ? "series_desc" : "series" ; ViewData[ "AuthorNameSortParm" ] = sortOrder == "authorname" ? "authorname_desc" : "authorname" ; ViewData[ "DateSortParm" ] = sortOrder == "date" ? "date_desc" : "date" ; if (searchString != null ) { pageNumber = 1; } else { searchString = currentFilter; } ViewData[ "CurrentFilter" ] = searchString; List<Post> posts = _blogManager.GetAllPosts( null ); if (!String.IsNullOrEmpty(searchString)) { posts = posts.Where(p => p.Title.Contains(searchString) || p.Category.Contains(searchString) || p.AuthorName.Contains(searchString) || p.Series.Contains(searchString)).ToList(); } switch (sortOrder) { case "title" : posts = posts.OrderBy(p => p.Title).ToList(); break ; case "title_desc" : posts = posts.OrderByDescending(p => p.Title).ToList(); break ; case "category" : posts = posts.OrderBy(p => p.Category).ToList(); break ; case "category_desc" : posts = posts.OrderByDescending(p => p.Category).ToList(); break ; case "series" : posts = posts.OrderBy(p => p.Series).ToList(); break ; case "series_desc" : posts = posts.OrderByDescending(p => p.Series).ToList(); break ; case "authorname" : posts = posts.OrderBy(p => p.AuthorName).ToList(); break ; case "authorname_desc" : posts = posts.OrderByDescending(p => p.AuthorName).ToList(); break ; case "date" : posts = posts.OrderBy(p => p.PublishedDate).ToList(); break ; case "date_desc" : posts = posts.OrderByDescending(p => p.PublishedDate).ToList(); break ; default : posts = posts.OrderByDescending(p => p.PublishedDate).ToList(); break ; } int pageSize = 5; return View(await Task.FromResult(PaginatedList<PostViewModel>.Create(_mapper.Map<List<PostViewModel>>(posts), pageNumber ?? 1, pageSize))); } [Authorize] // GET: BlogController/Create public ActionResult Create() { PostViewModel vm = new PostViewModel(); return View(vm); } [Authorize] // POST: BlogController/Create [HttpPost] [ValidateAntiForgeryToken] public ActionResult Create(PostViewModel model) { try { if (ModelState.IsValid) { _blogManager.Create(model); return RedirectToAction(nameof(Dashboard)); } return View(model); } catch (Exception ex) { return View(model); } } [Authorize] // GET: BlogController/Edit/5 public ActionResult Edit( string url) { var post = _blogManager.GetPostByUrl(url); if (post == null ) post = _blogManager.GetPostByUrlForArchival(url); if (post != null ) { PostViewModel model = _mapper.Map<PostViewModel>(post); model.Content = _blogManager.GetContentByUrl(url); return View(model); } return View(); } [Authorize] // POST: BlogController/Edit/5 [HttpPost] [ValidateAntiForgeryToken] public ActionResult Edit(PostViewModel model) { try { if (ModelState.IsValid) { _blogManager.Edit(model); return RedirectToAction(nameof(Dashboard)); } return View(model); } catch (Exception ex) { return View(model); } } [Authorize] // POST: BlogController/Delete/5 public ActionResult Archive( string url) { try { PostViewModel postVM = _mapper.Map<PostViewModel>(_blogManager.GetPostByUrlForArchival(url)); postVM.IsActive = !postVM.IsActive; _blogManager.Edit(postVM); return RedirectToAction(nameof(Dashboard)); } catch { return View(); } } } } |
HomeController.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 | using System; using System.Collections.Generic; using System.Linq; using System.Threading.Tasks; using AutoMapper; using Blog.BAL.Helpers; using Blog.BAL.Interfaces; using Blog.BAL.Models; using Blog.DAL.Entities; using Microsoft.AspNetCore.Authorization; using Microsoft.AspNetCore.Http; using Microsoft.AspNetCore.Mvc; namespace Blog.Web.Controllers { public class BlogController : Controller { private readonly IBlogManager _blogManager; private readonly IUserManager _userManager; private readonly ILdObjectManager _ldObjectManager; private readonly IHttpContextAccessor _httpContextAccessor; private readonly IMapper _mapper; public BlogController(IBlogManager blogerManager, IMapper mapper, IUserManager userManager, ILdObjectManager ldObjectManager, IHttpContextAccessor httpContextAccessor) { _blogManager = blogerManager; _userManager = userManager; _mapper = mapper; _ldObjectManager = ldObjectManager; _httpContextAccessor = httpContextAccessor; } // GET: BlogController public async Task<IActionResult> Index( string currentFilter, string searchString, string tagFilter, string catFilter, int ? pageNumber) { var model = new BlogViewModel(); if (searchString != null ) { pageNumber = 1; } else { searchString = currentFilter; } ViewData[ "CurrentFilter" ] = searchString; var posts = _blogManager.GetPosts( null ).OrderByDescending(p => p.PublishedDate).ToList(); var favPosts = posts.OrderByDescending(p => p.LikeCount).Take(5).ToList(); var popularPosts = posts.OrderByDescending(p => p.ViewCount).Take(5).ToList(); var aboutMe = _userManager.GetOwner(); model.Categories = posts.Select(p => p.Category).Distinct().ToList(); model.Categories.Add( "All" ); if (! string .IsNullOrEmpty(searchString)) { posts = posts.Where(p => p.Title.ToUpper().Contains(searchString.ToUpper()) || p.Category.ToUpper().Contains(searchString.ToUpper()) || p.AuthorName.ToUpper().Contains(searchString.ToUpper()) || p.Series.ToUpper().Contains(searchString.ToUpper()) || p.Preview.ToUpper().Contains(searchString.ToUpper()) || p.Tags.ToUpper().Contains(searchString.ToUpper())).ToList(); } if (! string .IsNullOrEmpty(tagFilter)) { ViewData[ "tagFilter" ] = tagFilter; posts = posts.Where(p => p.Tags.Contains(tagFilter)).ToList(); } ViewBag.LdJsonObject = _ldObjectManager.GetCarouselLdObject(posts); if (! string .IsNullOrEmpty(catFilter)) { var catUrl = $ "https://{_httpContextAccessor.HttpContext.Request.Host.Value}/?catFilter={catFilter}" ; ViewBag.LdJsonObject = _ldObjectManager.GetBreadcrumLdObject( new List< string > { "Blog" , catFilter }, new List< string > { url, catUrl }); ViewData[ "catFilter" ] = catFilter; posts = posts.Where(p => p.Category.ToUpper() == catFilter.ToUpper()).ToList(); } int pageSize = 10; model.PagedBlog = PaginatedList<PostViewModel>.Create(_mapper.Map<List<PostViewModel>>(posts), pageNumber ?? 1, pageSize); model.FavoritePosts = _mapper.Map<List<PostViewModel>>(favPosts); model.PopularPosts = _mapper.Map<List<PostViewModel>>(popularPosts); model.AboutMe = _mapper.Map<UserViewModel>(aboutMe); return View(await Task.FromResult(model)); } [Authorize] public async Task<IActionResult> Dashboard( string sortOrder, string currentFilter, string searchString, int ? pageNumber) { ViewData[ "TitleSortParm" ] = sortOrder == "title" ? "title_desc" : "title" ; ViewData[ "CategorySortParm" ] = sortOrder == "category" ? "category_desc" : "category" ; ViewData[ "SeriesSortParm" ] = sortOrder == "series" ? "series_desc" : "series" ; ViewData[ "AuthorNameSortParm" ] = sortOrder == "authorname" ? "authorname_desc" : "authorname" ; ViewData[ "DateSortParm" ] = sortOrder == "date" ? "date_desc" : "date" ; if (searchString != null ) { pageNumber = 1; } else { searchString = currentFilter; } ViewData[ "CurrentFilter" ] = searchString; List<Post> posts = _blogManager.GetAllPosts( null ); if (!String.IsNullOrEmpty(searchString)) { posts = posts.Where(p => p.Title.Contains(searchString) || p.Category.Contains(searchString) || p.AuthorName.Contains(searchString) || p.Series.Contains(searchString)).ToList(); } switch (sortOrder) { case "title" : posts = posts.OrderBy(p => p.Title).ToList(); break ; case "title_desc" : posts = posts.OrderByDescending(p => p.Title).ToList(); break ; case "category" : posts = posts.OrderBy(p => p.Category).ToList(); break ; case "category_desc" : posts = posts.OrderByDescending(p => p.Category).ToList(); break ; case "series" : posts = posts.OrderBy(p => p.Series).ToList(); break ; case "series_desc" : posts = posts.OrderByDescending(p => p.Series).ToList(); break ; case "authorname" : posts = posts.OrderBy(p => p.AuthorName).ToList(); break ; case "authorname_desc" : posts = posts.OrderByDescending(p => p.AuthorName).ToList(); break ; case "date" : posts = posts.OrderBy(p => p.PublishedDate).ToList(); break ; case "date_desc" : posts = posts.OrderByDescending(p => p.PublishedDate).ToList(); break ; default : posts = posts.OrderByDescending(p => p.PublishedDate).ToList(); break ; } int pageSize = 5; return View(await Task.FromResult(PaginatedList<PostViewModel>.Create(_mapper.Map<List<PostViewModel>>(posts), pageNumber ?? 1, pageSize))); } [Authorize] // GET: BlogController/Create public ActionResult Create() { PostViewModel vm = new PostViewModel(); return View(vm); } [Authorize] // POST: BlogController/Create [HttpPost] [ValidateAntiForgeryToken] public ActionResult Create(PostViewModel model) { try { if (ModelState.IsValid) { _blogManager.Create(model); return RedirectToAction(nameof(Dashboard)); } return View(model); } catch (Exception ex) { return View(model); } } [Authorize] // GET: BlogController/Edit/5 public ActionResult Edit( string url) { var post = _blogManager.GetPostByUrl(url); if (post == null ) post = _blogManager.GetPostByUrlForArchival(url); if (post != null ) { PostViewModel model = _mapper.Map<PostViewModel>(post); model.Content = _blogManager.GetContentByUrl(url); return View(model); } return View(); } [Authorize] // POST: BlogController/Edit/5 [HttpPost] [ValidateAntiForgeryToken] public ActionResult Edit(PostViewModel model) { try { if (ModelState.IsValid) { _blogManager.Edit(model); return RedirectToAction(nameof(Dashboard)); } return View(model); } catch (Exception ex) { return View(model); } } [Authorize] // POST: BlogController/Delete/5 public ActionResult Archive( string url) { try { PostViewModel postVM = _mapper.Map<PostViewModel>(_blogManager.GetPostByUrlForArchival(url)); postVM.IsActive = !postVM.IsActive; _blogManager.Edit(postVM); return RedirectToAction(nameof(Dashboard)); } catch { return View(); } } } } |
SitemapController.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 | using System; using System.Collections.Generic; using System.Linq; using System.Web; using AutoMapper; using Blog.BAL.Interfaces; using Blog.DAL.Entities; using Microsoft.AspNetCore.Http; using Microsoft.AspNetCore.Mvc; namespace Blog.Web.Controllers { public class SitemapController : Controller { private readonly IBlogManager _blogManager; private readonly IMapper _mapper; private readonly IHttpContextAccessor _httpContextAccessor; public SitemapController(IBlogManager blogerManager, IMapper mapper, IHttpContextAccessor httpContextAccessor) { _blogManager = blogerManager; _mapper = mapper; _httpContextAccessor = httpContextAccessor; } public IActionResult Index() { var posts = _blogManager.GetPosts( null ); var siteMapBuilder = new SitemapBuilder(); siteMapBuilder.AddUrl(baseUrl, modified: DateTime.UtcNow, changeFrequency: ChangeFrequency.Yearly, priority: 0.5); siteMapBuilder.AddUrl(baseUrl + "/AboutMe" , modified: DateTime.UtcNow, changeFrequency: ChangeFrequency.Yearly, priority: 0.6); siteMapBuilder.AddUrl(baseUrl + "/Blog" , modified: DateTime.UtcNow, changeFrequency: ChangeFrequency.Weekly, priority: 0.8); foreach (var cat in posts.Select(p => p.Category).Distinct().ToList()) { siteMapBuilder.AddUrl(baseUrl + "/Blog?catFilter=" + HttpUtility.UrlEncode(cat), modified: DateTime.UtcNow, changeFrequency: ChangeFrequency.Weekly, priority: 0.8); } foreach (var post in posts) { siteMapBuilder.AddUrl(baseUrl + "/?url=" + HttpUtility.UrlEncode(post.Content), modified: post.UpdatedOn, changeFrequency: ChangeFrequency.Weekly, priority: 1.0); siteMapBuilder.AddUrl(baseUrl + "/amp/?url=" + HttpUtility.UrlEncode(post.Content), modified: post.UpdatedOn, changeFrequency: ChangeFrequency.Weekly, priority: 1.0); } string xml = siteMapBuilder.ToString(); return Content(xml, "text/xml" ); } } } |
2. In Infrastructures folder, create below classes
AutoMapping.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 | using AutoMapper; using Blog.BAL.Models; using Blog.DAL.Entities; using System.Linq; using System.Web; public class AutoMapping : Profile { public AutoMapping() { CreateMap<User, UserViewModel>(); CreateMap<User, EditAboutMeViewModel>(); CreateMap<Skill, SkillViewModel>(); CreateMap<Experience, ExperienceViewModel>(); CreateMap<Education, EducationViewModel>(); CreateMap<Project, ProjectViewModel>(); CreateMap<ProjectLink, ProjectLinkViewModel>(); CreateMap<ProjectLinks, ProjectLinksViewModel>(); CreateMap<Post, PostViewModel>() .ForMember(vm => vm.TagsString, m => m.MapFrom(p => p.Tags)) .AfterMap((dto, jobDto) => jobDto.Tags = dto.Tags.Split( ',' ).ToList()); } }<br> |
PaginatedList.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 | using Microsoft.EntityFrameworkCore; using System; using System.Collections.Generic; using System.Linq; using System.Threading.Tasks; namespace Blog.Web.Infrastructures { public class PaginatedList<T> : List<T> { public int PageIndex { get ; private set ; } public int TotalPages { get ; private set ; } public PaginatedList(List<T> items, int count, int pageIndex, int pageSize) { PageIndex = pageIndex; TotalPages = ( int )Math.Ceiling(count / ( double )pageSize); this .AddRange(items); } public bool HasPreviousPage { get { return (PageIndex > 1); } } public bool HasNextPage { get { return (PageIndex < TotalPages); } } public static PaginatedList<T> Create(List<T> source, int pageIndex, int pageSize) { var count = source.Count(); var items = source.Skip((pageIndex - 1) * pageSize).Take(pageSize).ToList(); return new PaginatedList<T>(items, count, pageIndex, pageSize); } } } |
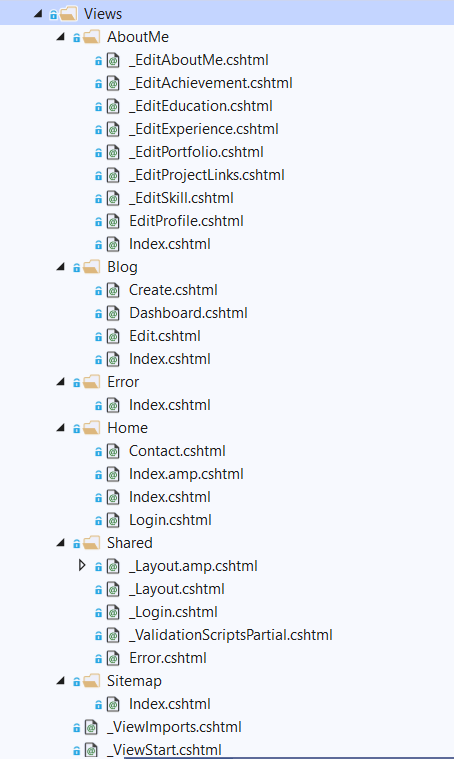
a. In AboutMe folder:
_EditAboutMe.cshtml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 | @model Blog.BAL.Models.EditAboutMeViewModel < div id = "abtMeContainer" > < form asp-action = "SaveAboutMe" id = "formAboutMe" enctype = "multipart/form-data" > < input name = "IsAboutMeValid" type = "hidden" value = "@ViewData.ModelState.IsValid.ToString()" /> < div class = "row" > < div class = "col-6" > < div class = "form-group" > < label asp-for = "FormFile" class = "control-label" ></ label > < div class = "custom-file mb-3" > @*< input asp-for = "EditAboutMe.FormFile" type = "file" class = "custom-file-input" id = "customFile" name = "filename" > < label class = "custom-file-label" for = "customFile" >Choose file</ label >*@ < input asp-for = "FormFile" type = "file" id = "fileProfileImage" > </ div > < span asp-validation-for = "FormFile" class = "text-danger" ></ span > </ div > < div class = "form-group" > < label asp-for = "Name" class = "control-label" ></ label > < input asp-for = "Name" class = "form-control" /> < span asp-validation-for = "Name" class = "text-danger" ></ span > </ div > </ div > < div class = "col-6" > < div class = "form-group" > < label asp-for = "Summary" class = "control-label" ></ label > < textarea class = "form-control" asp-for = "Summary" rows = "5" ></ textarea > < span asp-validation-for = "Summary" class = "text-danger" ></ span > </ div > </ div > </ div > < br /> < div class = "row" > < div class = "col-12" > < div class = "form-group text-center" > < input type = "submit" value = "Save" class = "btn btn-primary" id = "btnSaveAboutMe" /> </ div > </ div > </ div > </ form > </ div > |
_EditAchievement.cshtml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 | @model Blog.BAL.Models.EditAchievementViewModel < div id = "achievementContainer" > < form id = "formAddAchievement" asp-action = "AddAchievement" > < input name = "IsAchievementValid" type = "hidden" value = "@ViewData.ModelState.IsValid.ToString()" /> < div class = "row row-eq-height" id = "mainAddAchievementFrm" > < div class = "col-10" > < div class = "form-group" > < label asp-for = "Achievement" class = "control-label" ></ label > < div class = "input-group" > < input type = "text" class = "form-control" asp-for = "Achievement" /> </ div > < span asp-validation-for = "Achievement" class = "text-danger" ></ span > </ div > </ div > < div class = "col-2" > < label class = "control-label" > </ label > < div class = "form-group text-center" > < button class = "btn btn-outline-secondary" type = "submit" id = "btnAddAchievement" >Add</ button > </ div > </ div > </ div > < div id = "divAchievements" > @if (Model.Achievements.Count > 0) { < table class = "table table-striped" id = "tblAchievements" > < thead > < tr > < th scope = "col" style = "width: 90%;" >Name</ th > < th scope = "col" style = "width: 10%;" >Action</ th > </ tr > </ thead > < tbody > @for (var i = 0; i < Model.Achievements.Count (); i++) { <tr> < td > < div class = "collapse show multi-collapse-achievement_@i" > < span >@Model.Achievements[i]</ span > < input type = "hidden" id = "achievementIndex" class = "form-control" value = "@i" /> </ div > < div class = "collapse multi-collapse-achievement_@i" > < input type = "text" id = "achievementName_@i" class = "form-control" asp-for = "@Model.Achievements[i]" onkeydown = "return event.keyCode != 13;" /> </ div > </ td > < td class = "align-middle" > < div class = "collapse show multi-collapse-achievement_@i" > < a class = "edit" title = "Edit" data-toggle = "collapse" data-target = ".multi-collapse-achievement_@i" aria-expanded = "false" > < i class = "fas fa-edit" ></ i > </ a > < a class = "delete" title = "Delete" id = "btnRemoveAchievement" data-toggle = "tooltip" > < i class = "far fa-trash-alt" ></ i > </ a > < br /> </ div > < div class = "collapse multi-collapse-achievement_@i" > < a class = "update" title = "Update" id = "btnUpdateAchievement" data-toggle = "tooltip" > < i class = "far fa-check-circle" ></ i > </ a > < a class = "cancel" title = "Cancel" data-toggle = "collapse" data-target = ".multi-collapse-achievement_@i" aria-expanded = "false" > < i class = "far fa-times-circle" ></ i > </ a > </ div > </ td > </ tr > } </ tbody > </ table > < div class = "row" > < div class = "col-12" > < div class = "form-group text-center" > < input type = "submit" value = "Save" class = "btn btn-primary" id = "btnSaveAchievements" /> </ div > </ div > </ div > } </ div > </ form > </ div > |
_EditEducation.cshtml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 | @model Blog.BAL.Models.EditEducationViewModel < div id = "eduContainer" > < form id = "formAddEdu" asp-action = "AddEdu" > < input name = "IsEduValid" type = "hidden" value = "@ViewData.ModelState.IsValid.ToString()" /> < div id = "mainAddEduFrm" > < div class = "row row-eq-height" > < div class = "col-6" > < div class = "form-group" > < label asp-for = "School" class = "control-label" ></ label > < div class = "input-group" > < input type = "text" class = "form-control" asp-for = "School" > </ div > < span asp-validation-for = "School" class = "text-danger" ></ span > </ div > </ div > < div class = "col-6" > < div class = "form-group" > < label asp-for = "Major" class = "control-label" ></ label > < div class = "input-group" > < input type = "text" class = "form-control" asp-for = "Major" > </ div > < span asp-validation-for = "Major" class = "text-danger" ></ span > </ div > </ div > </ div > < div class = "row row-eq-height" > < div class = "col-6" > < div class = "form-group" > < label asp-for = "Location" class = "control-label" ></ label > < div class = "input-group" > < input type = "text" class = "form-control" asp-for = "Location" > </ div > < span asp-validation-for = "Location" class = "text-danger" ></ span > </ div > </ div > < div class = "col-6" > < div class = "form-group" > < label asp-for = "Duration" class = "control-label" ></ label > < div class = "input-group" > < input type = "text" class = "form-control" asp-for = "Duration" > </ div > < span asp-validation-for = "Duration" class = "text-danger" ></ span > </ div > </ div > </ div > < div class = "row" > < div class = "col-12" > < div class = "form-group" > < label asp-for = "Items" class = "control-label" ></ label > < textarea class = "form-control" asp-for = "Items" rows = "5" ></ textarea > < span asp-validation-for = "Items" class = "text-danger" ></ span > </ div > </ div > </ div > < div class = "row" > < div class = "col-12 text-center" > < div class = "form-group text-center" > < button class = "btn btn-outline-secondary" type = "submit" id = "btnAddEdu" >Add</ button > </ div > </ div > </ div > </ div > < div id = "divEdus" > @if (Model.Educations.Count > 0) { < table class = "table table-striped" id = "tblEducations" > < thead > < tr > < th scope = "col" style = "width: 90%;" >Educations</ th > < th scope = "col" style = "width: 10%;" >Action</ th > </ tr > </ thead > < tbody > @for (var i = 0; i < Model.Educations.Count (); i++) { <tr> < td > < div class = "row row-eq-height" > < div class = "col-6" > < div class = "form-group collapse show multi-collapse-edu_@i" > < label asp-for = "@Model.Educations[i].School" class = "control-label font-weight-bold" ></ label > < div class = "input-group pl-1" > < span >@Model.Educations[i].School</ span > < input type = "hidden" id = "eduIndex" class = "form-control" value = "@i" /> </ div > </ div > < div class = "form-group collapse multi-collapse-edu_@i" > < label asp-for = "@Model.Educations[i].School" class = "control-label" ></ label > < div class = "input-group" > < input type = "text" class = "form-control" asp-for = "@Model.Educations[i].School" > </ div > < span asp-validation-for = "@Model.Educations[i].School" class = "text-danger" ></ span > </ div > </ div > < div class = "col-6" > < div class = "form-group collapse show multi-collapse-edu_@i" > < label asp-for = "@Model.Educations[i].Major" class = "control-label font-weight-bold" ></ label > < div class = "input-group pl-1" > < span >@Model.Educations[i].Major</ span > </ div > </ div > < div class = "form-group collapse multi-collapse-edu_@i" > < label asp-for = "@Model.Educations[i].Major" class = "control-label" ></ label > < div class = "input-group" > < input type = "text" class = "form-control" asp-for = "@Model.Educations[i].Major" > </ div > < span asp-validation-for = "@Model.Educations[i].Major" class = "text-danger" ></ span > </ div > </ div > </ div > < div class = "row row-eq-height" > < div class = "col-6" > < div class = "form-group collapse show multi-collapse-edu_@i" > < label asp-for = "@Model.Educations[i].Location" class = "control-label font-weight-bold" ></ label > < div class = "input-group pl-1" > < span >@Model.Educations[i].Location</ span > </ div > </ div > < div class = "form-group collapse multi-collapse-edu_@i" > < label asp-for = "@Model.Educations[i].Location" class = "control-label" ></ label > < div class = "input-group" > < input type = "text" class = "form-control" asp-for = "@Model.Educations[i].Location" > </ div > < span asp-validation-for = "@Model.Educations[i].Location" class = "text-danger" ></ span > </ div > </ div > < div class = "col-6" > < div class = "form-group collapse show multi-collapse-edu_@i" > < label asp-for = "@Model.Educations[i].Duration" class = "control-label font-weight-bold" ></ label > < div class = "input-group" > < span >@Model.Educations[i].Duration</ span > </ div > </ div > < div class = "form-group collapse multi-collapse-edu_@i" > < label asp-for = "@Model.Educations[i].Duration" class = "control-label" ></ label > < div class = "input-group pl-1" > < input type = "text" class = "form-control" asp-for = "@Model.Educations[i].Duration" > </ div > < span asp-validation-for = "@Model.Educations[i].Duration" class = "text-danger" ></ span > </ div > </ div > </ div > < div class = "row" > < div class = "col-12" > < div class = "form-group collapse show multi-collapse-edu_@i" > < label asp-for = "@Model.Educations[i].Items" class = "control-label font-weight-bold" ></ label > < div class = "input-group pl-1" > < span style = "white-space:pre-wrap;" >@Model.Educations[i].Items</ span > </ div > </ div > < div class = "form-group collapse multi-collapse-edu_@i" > < label asp-for = "@Model.Educations[i].Items" class = "control-label" ></ label > < textarea class = "form-control" asp-for = "@Model.Educations[i].Items" rows = "5" ></ textarea > < span asp-validation-for = "@Model.Educations[i].Items" class = "text-danger" ></ span > </ div > </ div > </ div > </ td > < td class = "align-middle" > < div class = "collapse show multi-collapse-edu_@i" > < a class = "edit" title = "Edit" data-toggle = "collapse" data-target = ".multi-collapse-edu_@i" aria-expanded = "false" > < i class = "fas fa-edit" ></ i > </ a > < a class = "delete" title = "Delete" id = "btnRemoveEdu" data-toggle = "tooltip" > < i class = "far fa-trash-alt" ></ i > </ a > < br /> </ div > < div class = "collapse multi-collapse-edu_@i" > < a class = "update" title = "Update" id = "btnUpdateEdu" data-toggle = "tooltip" > < i class = "far fa-check-circle" ></ i > </ a > < a class = "cancel" title = "Cancel" data-toggle = "collapse" data-target = ".multi-collapse-edu_@i" aria-expanded = "false" > < i class = "far fa-times-circle" ></ i > </ a > </ div > </ td > </ tr > } </ tbody > </ table > < div class = "row" > < div class = "col-12" > < div class = "form-group text-center" > < input type = "submit" value = "Save" class = "btn btn-primary" id = "btnSaveEducations" /> </ div > </ div > </ div > } </ div > </ form > </ div > |
_EditExperience.cshtml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 | @model Blog.BAL.Models.EditExperienceViewModel < div id = "expContainer" > < form id = "formAddExp" asp-action = "AddExp" > < input name = "IsExpValid" type = "hidden" value = "@ViewData.ModelState.IsValid.ToString()" /> < div id = "mainAddExpFrm" > < div class = "row row-eq-height" > < div class = "col-6" > < div class = "form-group" > < label asp-for = "Title" class = "control-label" ></ label > < div class = "input-group" > < input type = "text" class = "form-control" asp-for = "Title" > </ div > < span asp-validation-for = "Title" class = "text-danger" ></ span > </ div > </ div > < div class = "col-6" > < div class = "form-group" > < label asp-for = "Company" class = "control-label" ></ label > < div class = "input-group" > < input type = "text" class = "form-control" asp-for = "Company" > </ div > < span asp-validation-for = "Company" class = "text-danger" ></ span > </ div > </ div > </ div > < div class = "row row-eq-height" > < div class = "col-6" > < div class = "form-group" > < label asp-for = "Location" class = "control-label" ></ label > < div class = "input-group" > < input type = "text" class = "form-control" asp-for = "Location" > </ div > < span asp-validation-for = "Location" class = "text-danger" ></ span > </ div > </ div > < div class = "col-6" > < div class = "form-group" > < label asp-for = "Duration" class = "control-label" ></ label > < div class = "input-group" > < input type = "text" class = "form-control" asp-for = "Duration" > </ div > < span asp-validation-for = "Duration" class = "text-danger" ></ span > </ div > </ div > </ div > < div class = "row" > < div class = "col-12" > < div class = "form-group" > < label asp-for = "Items" class = "control-label" ></ label > < textarea class = "form-control" asp-for = "Items" rows = "5" ></ textarea > < span asp-validation-for = "Items" class = "text-danger" ></ span > </ div > </ div > </ div > < div class = "row" > < div class = "col-12 text-center" > < div class = "form-group text-center" > < button class = "btn btn-outline-secondary" type = "submit" id = "btnAddExp" >Add</ button > </ div > </ div > </ div > </ div > < div id = "divExps" > @if (Model.Experiences.Count > 0) { < table class = "table table-striped" id = "tblExperiences" > < thead > < tr > < th scope = "col" style = "width: 90%;" >Experiences</ th > < th scope = "col" style = "width: 10%;" >Action</ th > </ tr > </ thead > < tbody > @for (var i = 0; i < Model.Experiences.Count (); i++) { <tr> < td > < div class = "row row-eq-height" > < div class = "col-6" > < div class = "form-group collapse show multi-collapse-exp_@i" > < label asp-for = "@Model.Experiences[i].Title" class = "control-label font-weight-bold" ></ label > < div class = "input-group pl-1" > < span >@Model.Experiences[i].Title</ span > < input type = "hidden" id = "expIndex" class = "form-control" value = "@i" /> </ div > </ div > < div class = "form-group collapse multi-collapse-exp_@i" > < label asp-for = "@Model.Experiences[i].Title" class = "control-label" ></ label > < div class = "input-group" > < input type = "text" class = "form-control" asp-for = "@Model.Experiences[i].Title" > </ div > < span asp-validation-for = "@Model.Experiences[i].Title" class = "text-danger" ></ span > </ div > </ div > < div class = "col-6" > < div class = "form-group collapse show multi-collapse-exp_@i" > < label asp-for = "@Model.Experiences[i].Company" class = "control-label font-weight-bold" ></ label > < div class = "input-group pl-1" > < span >@Model.Experiences[i].Company</ span > </ div > </ div > < div class = "form-group collapse multi-collapse-exp_@i" > < label asp-for = "@Model.Experiences[i].Company" class = "control-label" ></ label > < div class = "input-group" > < input type = "text" class = "form-control" asp-for = "@Model.Experiences[i].Company" > </ div > < span asp-validation-for = "@Model.Experiences[i].Company" class = "text-danger" ></ span > </ div > </ div > </ div > < div class = "row row-eq-height" > < div class = "col-6" > < div class = "form-group collapse show multi-collapse-exp_@i" > < label asp-for = "@Model.Experiences[i].Location" class = "control-label font-weight-bold" ></ label > < div class = "input-group pl-1" > < span >@Model.Experiences[i].Location</ span > </ div > </ div > < div class = "form-group collapse multi-collapse-exp_@i" > < label asp-for = "@Model.Experiences[i].Location" class = "control-label" ></ label > < div class = "input-group" > < input type = "text" class = "form-control" asp-for = "@Model.Experiences[i].Location" > </ div > < span asp-validation-for = "@Model.Experiences[i].Location" class = "text-danger" ></ span > </ div > </ div > < div class = "col-6" > < div class = "form-group collapse show multi-collapse-exp_@i" > < label asp-for = "@Model.Experiences[i].Duration" class = "control-label font-weight-bold" ></ label > < div class = "input-group pl-1" > < span >@Model.Experiences[i].Duration</ span > </ div > </ div > < div class = "form-group collapse multi-collapse-exp_@i" > < label asp-for = "@Model.Experiences[i].Duration" class = "control-label" ></ label > < div class = "input-group" > < input type = "text" class = "form-control" asp-for = "@Model.Experiences[i].Duration" > </ div > < span asp-validation-for = "@Model.Experiences[i].Duration" class = "text-danger" ></ span > </ div > </ div > </ div > < div class = "row" > < div class = "col-12" > < div class = "form-group collapse show multi-collapse-exp_@i" > < label asp-for = "@Model.Experiences[i].Items" class = "control-label font-weight-bold" ></ label > < div class = "input-group pl-1" > < span style = "white-space:pre-wrap;" >@Model.Experiences[i].Items</ span > </ div > </ div > < div class = "form-group collapse multi-collapse-exp_@i" > < label asp-for = "@Model.Experiences[i].Items" class = "control-label" ></ label > < textarea class = "form-control" asp-for = "@Model.Experiences[i].Items" rows = "5" ></ textarea > < span asp-validation-for = "@Model.Experiences[i].Items" class = "text-danger" ></ span > </ div > </ div > </ div > </ td > < td class = "align-middle" > < div class = "collapse show multi-collapse-exp_@i" > < a class = "edit" title = "Edit" data-toggle = "collapse" data-target = ".multi-collapse-exp_@i" aria-expanded = "false" > < i class = "fas fa-edit" ></ i > </ a > < a class = "delete" title = "Delete" id = "btnRemoveExp" data-toggle = "tooltip" > < i class = "far fa-trash-alt" ></ i > </ a > < br /> </ div > < div class = "collapse multi-collapse-exp_@i" > < a class = "update" title = "Update" id = "btnUpdateExp" data-toggle = "tooltip" > < i class = "far fa-check-circle" ></ i > </ a > < a class = "cancel" title = "Cancel" data-toggle = "collapse" data-target = ".multi-collapse-exp_@i" aria-expanded = "false" > < i class = "far fa-times-circle" ></ i > </ a > </ div > </ td > </ tr > } </ tbody > </ table > < div class = "row" > < div class = "col-12" > < div class = "form-group text-center" > < input type = "submit" value = "Save" class = "btn btn-primary" id = "btnSaveExperiences" /> </ div > </ div > </ div > } </ div > </ form > </ div > |
_EditPortfolio.cshtml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 | @model Blog.BAL.Models.EditPortfolioViewModel < div id = "projectContainer" > < form id = "formAddProject" asp-action = "AddProject" > < input name = "IsProjectValid" type = "hidden" value = "@ViewData.ModelState.IsValid.ToString()" /> < div id = "mainAddPrjFrm" > < div class = "row" > < div class = "col-6" > < div class = "form-group" > < label asp-for = "FormFile" class = "control-label" ></ label > < div class = "custom-file mb-3" > < input asp-for = "FormFile" type = "file" id = "fileProjectImage" > </ div > < span asp-validation-for = "FormFile" class = "text-danger" ></ span > </ div > </ div > < div class = "col-6" > < div class = "form-group" > < label asp-for = "Name" class = "control-label" ></ label > < input asp-for = "Name" class = "form-control" /> < span asp-validation-for = "Name" class = "text-danger" ></ span > </ div > </ div > </ div > < div class = "row" > < div class = "col-12" > < div class = "form-group" > < label asp-for = "Description" class = "control-label" ></ label > < textarea class = "form-control" asp-for = "Description" rows = "5" ></ textarea > < span asp-validation-for = "Description" class = "text-danger" ></ span > </ div > </ div > </ div > < div class = "row" > < div class = "col-12" > < button type = "button" class = "btn btn-link p-0 m-0 mb-2" id = "btnAddProjectLink" > Add Project Links </ button > </ div > </ div > < div class = "row" > < div class = "col-12" id = "mainAddProjectLink_" > @Html.Partial("_EditProjectLinks.cshtml", Model.Links) </ div > </ div > < div class = "row" > < div class = "col-12 text-center" > < div class = "form-group text-center" > < button class = "btn btn-outline-secondary" type = "submit" id = "btnAddProject" >Add</ button > </ div > </ div > </ div > </ div > < div id = "divPortfolios" > @if (Model.Projects.Count > 0) { < table class = "table table-striped" id = "tblEducations" > < thead > < tr > < th scope = "col" style = "width: 90%;" >Projects</ th > < th scope = "col" style = "width: 10%;" >Action</ th > </ tr > </ thead > < tbody > @for (var i = 0; i < Model.Projects.Count (); i++) { <tr> < td > < div class = "row row-eq-height" > < div class = "col-6" > < div class = "form-group collapse show multi-collapse-prj_@i" > < label asp-for = "@Model.Projects[i].Image" class = "control-label font-weight-bold" ></ label > < div class = "input-group pl-1" > @if (Blog.BAL.Helpers.Utility.IsBase64(Model.Projects[i].Image)) { < img src = '@String.Format("data:image/jpg;base64,{0}", Model.Projects[i].Image)' style = "width: 150px; height: 150px;" class = "img-responsive" /> } else { < img src = '/Uploads/ProjectImages/@Model.Projects[i].Image' style = "width: 150px; height: 150px;" class = "img-responsive" /> } < input type = "hidden" class = "form-control" asp-for = "@Model.Projects[i].Image" value = "@Model.Projects[i].Image" /> < input type = "hidden" class = "form-control" asp-for = "@Model.Projects[i].ImageFileName" value = "@Model.Projects[i].ImageFileName" /> < input type = "hidden" id = "prjIndex" name = "Project[@i]" class = "form-control" value = "@i" /> </ div > </ div > < div class = "form-group collapse multi-collapse-prj_@i" > < label asp-for = "@Model.Projects[i].FormFile" class = "control-label" ></ label > < div class = "custom-file mb-3" > < input asp-for = "@Model.Projects[i].FormFile" type = "file" id = "fileProjectImage_@i" > </ div > < span asp-validation-for = "@Model.Projects[i].FormFile" class = "text-danger" ></ span > </ div > </ div > < div class = "col-6" > < div class = "form-group collapse show multi-collapse-prj_@i" > < label asp-for = "@Model.Projects[i].Name" class = "control-label font-weight-bold" ></ label > < div class = "input-group pl-1" > < span >@Model.Projects[i].Name</ span > </ div > </ div > < div class = "form-group collapse multi-collapse-prj_@i" > < label asp-for = "@Model.Projects[i].Name" class = "control-label" ></ label > < div class = "input-group" > < input type = "text" class = "form-control" asp-for = "@Model.Projects[i].Name" > </ div > < span asp-validation-for = "@Model.Projects[i].Name" class = "text-danger" ></ span > </ div > </ div > </ div > < div class = "row row-eq-height" > < div class = "col-12" > < div class = "form-group collapse show multi-collapse-prj_@i" > < label asp-for = "@Model.Projects[i].Description" class = "control-label font-weight-bold" ></ label > < div class = "input-group pl-1" > < span >@Model.Projects[i].Description</ span > </ div > </ div > < div class = "form-group collapse multi-collapse-prj_@i" > < label asp-for = "@Model.Projects[i].Description" class = "control-label" ></ label > < textarea class = "form-control" asp-for = "@Model.Projects[i].Description" rows = "5" ></ textarea > < span asp-validation-for = "Description" class = "text-danger" ></ span > </ div > </ div > </ div > < div class = "row collapse multi-collapse-prj_@i" > < div class = "col-12" > < button type = "button" class = "btn btn-link p-0 m-0 mb-2" id = "btnAddProjectLink" > Add Project Links </ button > </ div > </ div > < div class = "row row-eq-height collapse multi-collapse-prj_@i" > < div class = "col-12" id = "mainAddProjectLink_@i" > @Html.Partial("_EditProjectLinks.cshtml", Model.Projects[i].Links) </ div > </ div > < div class = "row row-eq-height collapse show multi-collapse-prj_@i" > < div class = "col-12" > @if (Model.Projects[i].Links.Links.Count > 0) { < table class = "table" id = "tblProjects" > < thead > < tr > < th scope = "col" style = "width: 40%;" >Link Name</ th > < th scope = "col" style = "width: 40%;" >Link</ th > < th class = "collapse multi-collapse-prj_@i" scope = "col" style = "width: 20%;" >Action</ th > </ tr > </ thead > < tbody > @for (var j = 0; j < Model.Projects [i].Links.Links.Count(); j++) { <tr> < td > < div > @*class="collapse show multi-collapse-prj_@i">*@ < span >@Model.Projects[i].Links.Links[j].LinkName</ span > </ div > @*< div class = "collapse multi-collapse-prj_@i" > < input type = "text" id = "prjLinkName_@j" class = "form-control" asp-for = "@Model.Projects[i].Links.Links[j].LinkName" onkeydown = "return event.keyCode != 13;" /> </ div >*@ </ td > < td > < div > @*class="collapse show multi-collapse-prj_@i">*@ < span >@Model.Projects[i].Links.Links[j].Link</ span > </ div > @*< div class = "collapse multi-collapse-prj_@i" > < input type = "text" id = "prjLink_@j" class = "form-control" asp-for = "@Model.Projects[i].Links.Links[j].Link" onkeydown = "return event.keyCode != 13;" /> </ div >*@ </ td > @*< td class = "collapse multi-collapse-prj_@i" > < div class = "form-group" > < button type = "button" class = "btn btn-danger" id = "btnRemoveProjectLink" > Remove </ button > </ div > </ td >*@ </ tr > } </ tbody > </ table > } </ div > </ div > </ td > < td class = "align-middle" > < div class = "collapse show multi-collapse-prj_@i" > < a class = "edit" title = "Edit" data-toggle = "collapse" data-target = ".multi-collapse-prj_@i" aria-expanded = "false" > < i class = "fas fa-edit" ></ i > </ a > < a class = "delete" title = "Delete" id = "btnRemoveProject" data-toggle = "tooltip" > < i class = "far fa-trash-alt" ></ i > </ a > < br /> </ div > < div class = "collapse multi-collapse-prj_@i" > < a class = "update" title = "Update" id = "btnUpdateProject" data-toggle = "tooltip" > < i class = "far fa-check-circle" ></ i > </ a > < a class = "cancel" title = "Cancel" data-toggle = "collapse" data-target = ".multi-collapse-prj_@i" aria-expanded = "false" > < i class = "far fa-times-circle" ></ i > </ a > </ div > </ td > </ tr > } </ tbody > </ table > < div class = "row" > < div class = "col-12" > < div class = "form-group text-center" > < input type = "submit" value = "Save" class = "btn btn-primary" id = "btnSavePortfolios" /> </ div > </ div > </ div > } </ div > </ form > </ div > |
_EditProjectLinks.cshtml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 | @model Blog.BAL.Models.ProjectLinksViewModel < div id = "projectLinkContainer" > < form id = "formAddProjectLink" asp-action = "AddProjectLink" > < input name = "IsProjectLinkValid" type = "hidden" value = "@ViewData.ModelState.IsValid.ToString()" /> < div > @for (var i = 0; i < Model.Links.Count (); i++) { <div class = "row" > < div class = "col-5" > < div class = "col-5" > < div class = "form-group" > < label asp-for = "@Model.Links[i].LinkName" class = "control-label" ></ label > < input asp-for = "@Model.Links[i].LinkName" class = "form-control" /> < span asp-validation-for = "@Model.Links[i].LinkName" class = "text-danger" ></ span > </ div > </ div > < input type = "hidden" id = "prjLinkIndex" name = "prjLinkIndex_@i" class = "form-control" value = "@i" /> < div class = "form-group" > < label asp-for = "@Model.Links[i].Link" class = "control-label" ></ label > < input asp-for = "@Model.Links[i].Link" class = "form-control" /> < span asp-validation-for = "@Model.Links[i].Link" class = "text-danger" ></ span > </ div > </ div > < div class = "col-2" > < label class = "control-label" > </ label > < div class = "form-group" > < button type = "button" class = "btn btn-danger" id = "btnRemoveProjectLink" > Remove </ button > </ div > </ div > </ div > } </ div > </ form > </ div > |
_EditSkill.cshtml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 | @model Blog.BAL.Models.EditSkillViewModel < div id = "skillContainer" > < form id = "formAddSkill" asp-action = "AddSkill" > < input name = "IsSkillValid" type = "hidden" value = "@ViewData.ModelState.IsValid.ToString()" /> < div class = "row row-eq-height" id = "mainAddSkillFrm" > < div class = "col-3" > < div class = "form-group" > < label asp-for = "Category" class = "control-label" ></ label > < div class = "input-group" > < input id = "newSkillName" type = "text" class = "form-control" asp-for = "Category" > </ div > < span asp-validation-for = "Category" class = "text-danger" ></ span > </ div > </ div > < div class = "col-3" > < div class = "form-group" > < label asp-for = "Name" class = "control-label" ></ label > < div class = "input-group" > < input id = "newSkillName" type = "text" class = "form-control" asp-for = "Name" > </ div > < span asp-validation-for = "Name" class = "text-danger" ></ span > </ div > </ div > < div class = "col-3" > < div class = "form-group" > < label asp-for = "StartYear" class = "control-label" ></ label > < div class = "input-group" > < input id = "newSkillStartYear" type = "text" class = "form-control" asp-for = "StartYear" > </ div > < span asp-validation-for = "StartYear" class = "text-danger" ></ span > </ div > </ div > < div class = "col-3 text-center" > < label class = "control-label" > </ label > < div class = "form-group text-center" > < button class = "btn btn-outline-secondary" type = "submit" id = "btnAddSkill" >Add</ button > </ div > </ div > </ div > < div id = "divSkills" > @if (Model.Skills.Count > 0) { < table class = "table table-striped" id = "tblSkills" > < thead > < tr > < th scope = "col" style = "width: 20%;" >Category</ th > < th scope = "col" style = "width: 20%;" >Name</ th > < th scope = "col" style = "width: 20%;" >Start Year</ th > < th scope = "col" style = "width: 20%;" >Rating</ th > < th scope = "col" style = "width: 20%;" >Action</ th > </ tr > </ thead > < tbody > @for (var i = 0; i < Model.Skills.Count (); i++) { <tr> < td > < div class = "collapse show multi-collapse_@i" > < span >@Model.Skills[i].Category</ span > < input type = "hidden" id = "skillIndex" class = "form-control" value = "@i" /> </ div > < div class = "collapse multi-collapse_@i" > < input type = "text" id = "skillName_@i" class = "form-control" asp-for = "@Model.Skills[i].Category" onkeydown = "return event.keyCode != 13;" /> </ div > < span asp-validation-for = "@Model.Skills[i].Category" class = "text-danger" ></ span > </ td > < td > < div class = "collapse show multi-collapse_@i" > < span >@Model.Skills[i].Name</ span > < input type = "hidden" id = "skillIndex" class = "form-control" value = "@i" /> </ div > < div class = "collapse multi-collapse_@i" > < input type = "text" id = "skillName_@i" class = "form-control" asp-for = "@Model.Skills[i].Name" onkeydown = "return event.keyCode != 13;" /> </ div > < span asp-validation-for = "@Model.Skills[i].Name" class = "text-danger" ></ span > </ td > < td > < div class = "collapse show multi-collapse_@i" > < span >@Model.Skills[i].StartYear</ span > </ div > < div class = "collapse multi-collapse_@i" > < input type = "text" id = "skillStartYear_@i" class = "form-control" asp-for = "@Model.Skills[i].StartYear" onkeydown = "return event.keyCode != 13;" /> </ div > < span asp-validation-for = "@Model.Skills[i].StartYear" class = "text-danger" ></ span > </ td > < td > < div > < span >@Model.Skills[i].Rating</ span > </ div > < div > < input type = "hidden" id = "skillRating_@i" class = "form-control" asp-for = "@Model.Skills[i].Rating" /> </ div > </ td > < td class = "align-middle" > < div class = "collapse show multi-collapse_@i" > < a class = "edit" title = "Edit" data-toggle = "collapse" data-target = ".multi-collapse_@i" aria-expanded = "false" > < i class = "fas fa-edit" ></ i > </ a > < a class = "delete" title = "Delete" id = "btnRemoveSkill" data-toggle = "tooltip" > < i class = "far fa-trash-alt" ></ i > </ a > < br /> </ div > < div class = "collapse multi-collapse_@i" > < a class = "update" title = "Update" id = "btnUpdateSkill" data-toggle = "tooltip" > < i class = "far fa-check-circle" ></ i > </ a > < a class = "cancel" title = "Cancel" data-toggle = "collapse" data-target = ".multi-collapse_@i" aria-expanded = "false" > < i class = "far fa-times-circle" ></ i > </ a > </ div > </ td > </ tr > } </ tbody > </ table > < div class = "row" > < div class = "col-12" > < div class = "form-group text-center" > < input type = "submit" value = "Save" class = "btn btn-primary" id = "btnSaveSkills" /> </ div > </ div > </ div > } </ div > </ form > </ div > |
EditProfile.cshtml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 369 370 371 372 373 374 375 376 377 378 379 380 381 382 383 384 385 386 387 388 389 390 391 392 393 394 395 396 397 398 399 400 401 402 403 404 405 406 407 408 409 410 411 412 413 414 415 416 417 418 419 420 421 422 423 424 425 426 427 428 429 430 431 432 433 434 435 436 437 438 439 440 441 442 443 444 445 446 447 448 449 450 451 452 453 454 455 456 457 458 459 460 461 462 463 464 465 466 467 468 469 470 471 472 473 474 475 476 477 478 479 480 481 482 483 484 485 486 487 488 489 490 491 492 493 494 495 496 497 498 499 500 501 502 503 504 505 506 507 508 509 510 511 512 513 514 515 516 517 518 519 520 521 522 523 524 525 526 527 528 529 530 531 532 533 534 535 536 537 538 539 540 541 542 543 544 545 546 547 548 549 550 551 552 553 554 555 556 557 558 559 560 561 562 563 564 565 566 567 568 569 570 571 572 573 574 575 576 577 578 579 580 581 582 583 584 585 586 587 588 589 590 591 592 593 594 595 596 597 598 599 600 601 602 603 604 605 606 607 608 | @model Blog.BAL.Models.EditUserViewModel @{ ViewData["Title"] = "Edit Profile"; } < div class = "container pt-3" > < div class = "card" style = "width: 100%;" > < h2 class = "card-header" >About Me</ h2 > < div class = "card-body" > < div class = "row" > < div class = "col-12" > @Html.Partial("_EditAboutMe.cshtml", Model.EditAboutMe) </ div > </ div > </ div > </ div > < br /> < div class = "card" style = "width: 100%;" > < h2 class = "card-header" >Skills</ h2 > < div class = "card-body" > < div class = "row" > < div class = "col-12" > @Html.Partial("_EditSkill.cshtml", Model.EditSkill) </ div > </ div > </ div > </ div > < br /> < div class = "card" style = "width: 100%;" > < h2 class = "card-header" >Experience and Leadership</ h2 > < div class = "card-body" > < div class = "row" > < div class = "col-12" > @Html.Partial("_EditExperience.cshtml", Model.EditExperience) </ div > </ div > </ div > </ div > < br /> < div class = "card" style = "width: 100%;" > < h2 class = "card-header" >Education</ h2 > < div class = "card-body" > < div class = "row" > < div class = "col-12" > @Html.Partial("_EditEducation.cshtml", Model.EditEducation) </ div > </ div > </ div > </ div > < br /> < div class = "card" style = "width: 100%;" > < h2 class = "card-header" >Achievements</ h2 > < div class = "card-body" > < div class = "row" > < div class = "col-12" > @Html.Partial("_EditAchievement.cshtml", Model.EditAchievement) </ div > </ div > </ div > </ div > < br /> < div class = "card" style = "width: 100%;" > < h2 class = "card-header" >Portfolios</ h2 > < div class = "card-body" > < div class = "row" > < div class = "col-12" > @Html.Partial("_EditPortfolio.cshtml", Model.EditPortfolio) </ div > </ div > </ div > </ div > </ div > @section Scripts { @{await Html.RenderPartialAsync("_ValidationScriptsPartial");} < script type = "text/javascript" > $(document).on('click', '#btnSaveAboutMe', function (event) { event.preventDefault(); var fdata = new FormData(); var fileInput = $('#fileProfileImage')[0]; var file = fileInput.files[0]; fdata.append("FormFile", file); $("input[type='text'").each(function (x, y) { fdata.append($(y).attr("name"), $(y).val()); }); $("textarea").each(function (x, y) { fdata.append($(y).attr("name"), $(y).val()); }); $.ajax({ async: true, data: fdata, type: 'POST', url: $('#formAboutMe').attr('action'), processData: false, contentType: false, success: function (partialView) { var newBody = $('#formAboutMe', partialView); var isValid = newBody.find('[name="IsAboutMeValid"]').val() == 'True'; if (isValid) { alert("About Me is successfully Saved!"); } $('#abtMeContainer').html(partialView); } }); }); $(document).on('click', '#btnSaveSkills', function (event) { event.preventDefault(); var data = $('#divSkills :input').serialize(); $.ajax({ async: true, data: data, type: 'POST', url: '/AboutMe/SaveSkills', success: function (partialView) { alert("Skills are successfully Saved!"); $('#skillContainer').html(partialView); } }); }); $(document).on('click', '#btnAddSkill', function (event) { event.preventDefault(); var data = $('#formAddSkill').serialize(); $.ajax({ async: true, data: data, type: 'POST', url: $('#formAddSkill').attr('action'), success: function (partialView) { $('#skillContainer').html(partialView); var newBody = $('#formAddSkill', partialView); var isValid = newBody.find('[name="IsSkillValid"]').val() == 'True'; if (isValid) { $("div#mainAddSkillFrm input[type=text]").val(""); } } }); }); $(document).on('click', '#btnRemoveSkill', function (event) { event.preventDefault(); var id = $(this).closest('tr').find("#skillIndex").val(); var data = $('#divSkills :input').serialize() + '&id=' + id; $.ajax({ async: true, data: data, type: 'POST', url: '/AboutMe/RemoveSkill', success: function (partialView) { $('#skillContainer').html(partialView); } }); }); $(document).on('click', '#btnUpdateSkill', function (event) { event.preventDefault(); var id = $(this).closest('tr').find("#skillIndex").val(); var data = $('#divSkills :input').serialize() + '&id=' + id; $.ajax({ async: true, data: data, type: 'POST', url: '/AboutMe/UpdateSkill', success: function (partialView) { $('#skillContainer').html(partialView); } }); }); $(document).on('click', '#btnSaveExperiences', function (event) { event.preventDefault(); var data = $('#divExps :input').serialize(); $.ajax({ async: true, data: data, type: 'POST', url: '/AboutMe/SaveExperiences', success: function (partialView) { alert("Experiences are successfully Saved!"); $('#expContainer').html(partialView); } }); }); $(document).on('click', '#btnAddExp', function (event) { event.preventDefault(); var data = $('#formAddExp').serializeArray(); $.ajax({ async: true, data: data, type: 'POST', url: $('#formAddExp').attr('action'), success: function (partialView) { $('#expContainer').html(partialView); var newBody = $('#formAddExp', partialView); var isValid = newBody.find('[name="IsExpValid"]').val() == 'True'; if (isValid) { $("div#mainAddExpFrm input[type=text]").val(""); $("div#mainAddExpFrm textarea").val(""); } } }); }); $(document).on('click', '#btnRemoveExp', function (event) { event.preventDefault(); var id = $(this).closest('tr').find("#expIndex").val(); var data = $('#divExps :input').serialize() + '&id=' + id; $.ajax({ async: true, data: data, type: 'POST', url: '/AboutMe/RemoveExp', success: function (partialView) { $('#expContainer').html(partialView); } }); }); $(document).on('click', '#btnUpdateExp', function (event) { event.preventDefault(); var id = $(this).closest('tr').find("#expIndex").val(); var data = $('#divExps :input').serialize() + '&id=' + id; $.ajax({ async: true, data: data, type: 'POST', url: '/AboutMe/UpdateExp', success: function (partialView) { $('#expContainer').html(partialView); } }); }); $(document).on('click', '#btnSaveEducations', function (event) { event.preventDefault(); var data = $('#divEdus :input').serialize(); $.ajax({ async: true, data: data, type: 'POST', url: '/AboutMe/SaveEducations', success: function (partialView) { alert("Educations are successfully Saved!"); $('#eduContainer').html(partialView); } }); }); $(document).on('click', '#btnAddEdu', function (event) { event.preventDefault(); var data = $('#formAddEdu').serializeArray(); $.ajax({ async: true, data: data, type: 'POST', url: $('#formAddEdu').attr('action'), success: function (partialView) { $('#eduContainer').html(partialView); var newBody = $('#formAddEdu', partialView); var isValid = newBody.find('[name="IsEduValid"]').val() == 'True'; if (isValid) { $("div#mainAddEduFrm input[type=text]").val(""); $("div#mainAddEduFrm textarea").val(""); } } }); }); $(document).on('click', '#btnRemoveEdu', function (event) { event.preventDefault(); var id = $(this).closest('tr').find("#eduIndex").val(); var data = $('#divEdus :input').serialize() + '&id=' + id; $.ajax({ async: true, data: data, type: 'POST', url: '/AboutMe/RemoveEdu', success: function (partialView) { $('#eduContainer').html(partialView); } }); }); $(document).on('click', '#btnUpdateEdu', function (event) { event.preventDefault(); var id = $(this).closest('tr').find("#eduIndex").val(); var data = $('#divEdus :input').serialize() + '&id=' + id; $.ajax({ async: true, data: data, type: 'POST', url: '/AboutMe/UpdateEdu', success: function (partialView) { $('#eduContainer').html(partialView); } }); }); $(document).on('click', '#btnSaveAchievements', function (event) { event.preventDefault(); var data = $('#divAchievements :input').serialize(); $.ajax({ async: true, data: data, type: 'POST', url: '/AboutMe/SaveAchievements', success: function (partialView) { alert("Achievements are successfully Saved!"); $('#achievementContainer').html(partialView); } }); }); $(document).on('click', '#btnAddAchievement', function (event) { event.preventDefault(); var data = $('#formAddAchievement').serializeArray(); $.ajax({ async: true, data: data, type: 'POST', url: $('#formAddAchievement').attr('action'), success: function (partialView) { $('#achievementContainer').html(partialView); var newBody = $('#formAddAchievement', partialView); var isValid = newBody.find('[name="IsAchievementValid"]').val() == 'True'; if (isValid) { $("div#mainAddAchievementFrm input[type=text]").val(""); } } }); }); $(document).on('click', '#btnRemoveAchievement', function (event) { event.preventDefault(); var id = $(this).closest('tr').find("#achievementIndex").val(); var data = $('#divAchievements :input').serialize() + '&id=' + id; $.ajax({ async: true, data: data, type: 'POST', url: '/AboutMe/RemoveAchievement', success: function (partialView) { $('#achievementContainer').html(partialView); $("div#mainAddAchievementFrm input[type=text]").val(""); } }); }); $(document).on('click', '#btnUpdateAchievement', function (event) { event.preventDefault(); var id = $(this).closest('tr').find("#achievementIndex").val(); var data = $('#divAchievements :input').serialize() + '&id=' + id; $.ajax({ async: true, data: data, type: 'POST', url: '/AboutMe/UpdateAchievement', success: function (partialView) { $('#achievementContainer').html(partialView); $("div#mainAddAchievementFrm input[type=text]").val(""); } }); }); $(document).on('click', '#btnAddProjectLink', function (event) { event.preventDefault(); var id = $(this).closest('tr').find("#prjIndex").val(); id = id == null ? '' : id; var data = $('#mainAddProjectLink_' + id + ' :input').serialize(); $.ajax({ async: true, data: data, type: 'POST', url: '/AboutMe/AddProjectLink', success: function (partialView) { $('#mainAddProjectLink_' + id).html(partialView); } }); }); $(document).on('click', '#btnRemoveProjectLink', function (event) { event.preventDefault(); var prjIndex = $(this).closest('tr').find("#prjIndex").val(); prjIndex = prjIndex == null ? '' : prjIndex; var id = $(this).closest('.row').find("#prjLinkIndex").val(); var data = $('#mainAddProjectLink_' + prjIndex + ' :input').serialize() + '&id=' + id; $.ajax({ async: true, data: data, type: 'POST', url: '/AboutMe/RemoveProjectLink', success: function (partialView) { $('#mainAddProjectLink_' + prjIndex).html(partialView); } }); }); $(document).on('click', '#btnAddProject', function (event) { event.preventDefault(); var fdata = new FormData(); var fileInput = $('#fileProjectImage')[0]; var file = fileInput.files[0]; fdata.append("FormFile", file); $("#projectContainer input[type='text'], #divPortfolios input[type='hidden']").each(function (x, y) { var prjIndex = $(this).closest('tr').find("#prjIndex").val(); prjIndex = prjIndex == null ? '' : prjIndex; if ($(y).attr("name").includes('Link') && prjIndex == '') fdata.append('Links.' + $(y).attr("name"), $(y).val()); else if ($(y).attr("name").includes('Link') && prjIndex != '') fdata.append('Projects[' + prjIndex + '].Links.' + $(y).attr("name"), $(y).val()); else fdata.append($(y).attr("name"), $(y).val()); }); $("#projectContainer textarea").each(function (x, y) { fdata.append($(y).attr("name"), $(y).val()); }); $.ajax({ async: true, data: fdata, type: 'POST', url: $('#formAddProject').attr('action'), processData: false, contentType: false, success: function (partialView) { $('#projectContainer').html(partialView); var newBody = $('#formAddProject', partialView); var isValid = newBody.find('[name="IsProjectValid"]').val() == 'True'; if (isValid) { $("div#mainAddPrjFrm input[type=text]").val(""); $("div#mainAddPrjFrm textarea").val(""); } } }); }); $(document).on('click', '#btnUpdateProject', function (event) { event.preventDefault(); var prjIndex = $(this).closest('tr').find("#prjIndex").val(); var fdata = new FormData(); $("#divPortfolios input[type='text'], #divPortfolios input[type='hidden']").each(function (x, y) { var prjIndex = $(this).closest('tr').find("#prjIndex").val(); prjIndex = prjIndex == null ? '' : prjIndex; var fileInput = $('#fileProjectImage_' + prjIndex)[0]; var file = fileInput.files[0]; fdata.append('Projects[' + prjIndex + '].FormFile', file); if ($(y).attr("name").includes('Link') && prjIndex == '') fdata.append('Links.' + $(y).attr("name"), $(y).val()); else if ($(y).attr("name").includes('Link') && prjIndex != '') fdata.append('Projects[' + prjIndex + '].Links.' + $(y).attr("name"), $(y).val()); else fdata.append($(y).attr("name"), $(y).val()); }); $("#divPortfolios textarea").each(function (x, y) { fdata.append($(y).attr("name"), $(y).val()); }); fdata.append('prjIndex', prjIndex) $.ajax({ async: true, data: fdata, type: 'POST', processData: false, contentType: false, url: '/AboutMe/UpdateProject', success: function (partialView) { $('#projectContainer').html(partialView); } }); }); $(document).on('click', '#btnRemoveProject', function (event) { event.preventDefault(); var prjIndex = $(this).closest('tr').find("#prjIndex").val(); var fdata = new FormData(); $("#divPortfolios input[type='text'], #divPortfolios input[type='hidden']").each(function (x, y) { var prjIndex = $(this).closest('tr').find("#prjIndex").val(); prjIndex = prjIndex == null ? '' : prjIndex; var fileInput = $('#fileProjectImage_' + prjIndex)[0]; var file = fileInput.files[0]; fdata.append('Projects[' + prjIndex + '].FormFile', file); if ($(y).attr("name").includes('Link') && prjIndex == '') fdata.append('Links.' + $(y).attr("name"), $(y).val()); else if ($(y).attr("name").includes('Link') && prjIndex != '') fdata.append('Projects[' + prjIndex + '].Links.' + $(y).attr("name"), $(y).val()); else fdata.append($(y).attr("name"), $(y).val()); }); $("#divPortfolios textarea").each(function (x, y) { fdata.append($(y).attr("name"), $(y).val()); }); fdata.append('prjIndex', prjIndex) $.ajax({ async: true, data: fdata, type: 'POST', processData: false, contentType: false, url: '/AboutMe/RemoveProject', success: function (partialView) { $('#projectContainer').html(partialView); } }); }); $(document).on('click', '#btnSavePortfolios', function (event) { event.preventDefault(); var fdata = new FormData(); $("#divPortfolios input[type='text'], #divPortfolios input[type='hidden']").each(function (x, y) { var prjIndex = $(this).closest('tr').find("#prjIndex").val(); prjIndex = prjIndex == null ? '' : prjIndex; var fileInput = $('#fileProjectImage_' + prjIndex)[0]; var file = fileInput.files[0]; fdata.append('Projects[' + prjIndex + '].FormFile', file); if ($(y).attr("name").includes('Link') && prjIndex == '') fdata.append('Links.' + $(y).attr("name"), $(y).val()); else if ($(y).attr("name").includes('Link') && prjIndex != '') fdata.append('Projects[' + prjIndex + '].Links.' + $(y).attr("name"), $(y).val()); else fdata.append($(y).attr("name"), $(y).val()); }); $("#divPortfolios textarea").each(function (x, y) { fdata.append($(y).attr("name"), $(y).val()); }); $.ajax({ async: true, data: fdata, type: 'POST', processData: false, contentType: false, url: '/AboutMe/SavePortfolios', success: function (partialView) { alert("Projects are successfully Saved!"); $('#projectContainer').html(partialView); } }); }); </ script > } |
b. In Blog folder:
Create.cshtml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 | @model Blog.BAL.Models.PostViewModel @{ ViewData["Title"] = "Compose Post"; } < div class = "container" > < br /> < div class = "pb-3" > < a asp-action = "Dashboard" >Back to Dashboard</ a > </ div > < form asp-action = "Create" enctype = "multipart/form-data" > < div class = "row" > < div class = "col-4" > < div class = "form-group" > < label asp-for = "Title" class = "control-label" ></ label > < input asp-for = "Title" class = "form-control" /> < input type = "hidden" asp-for = "IsActive" value = "true" /> < input type = "hidden" asp-for = "Preview" /> < span asp-validation-for = "Title" class = "text-danger" ></ span > </ div > </ div > < div class = "col-4" > < label asp-for = "AuthorName" class = "control-label" ></ label > < input asp-for = "AuthorName" class = "form-control" /> < span asp-validation-for = "AuthorName" class = "text-danger" ></ span > </ div > < div class = "col-4" > < div class = "form-group" > < label asp-for = "FormFile" class = "control-label" ></ label > < div class = "custom-file mb-3" > < input asp-for = "FormFile" type = "file" id = "filePostThumbnail" > </ div > < span asp-validation-for = "FormFile" class = "text-danger" ></ span > </ div > </ div > </ div > < div class = "row" > < div class = "col-4" > < label asp-for = "Category" class = "control-label" ></ label > < input asp-for = "Category" class = "form-control" /> < span asp-validation-for = "Category" class = "text-danger" ></ span > </ div > < div class = "col-4" > < label asp-for = "Series" class = "control-label" ></ label > < input asp-for = "Series" class = "form-control" /> < span asp-validation-for = "Series" class = "text-danger" ></ span > </ div > < div class = "col-4" > < label asp-for = "PublishedDate" class = "control-label" ></ label > < input asp-for = "PublishedDate" class = "form-control" /> < span asp-validation-for = "PublishedDate" class = "text-danger" ></ span > </ div > </ div > < div class = "row pt-3" > < div class = "col-12" > < label asp-for = "Content" class = "control-label" ></ label > < textarea asp-for = "Content" class = "form-control" rows = "5" ></ textarea > < span asp-validation-for = "Content" class = "text-danger" ></ span > </ div > </ div > < div class = "row pt-3" > < div class = "col-12" > < label asp-for = "TagsString" class = "control-label" ></ label > < input asp-for = "TagsString" class = "form-control" /> < span asp-validation-for = "TagsString" class = "text-danger" ></ span > </ div > </ div > < div class = "row pt-2" > < div class = "col-12" > < div class = "form-group text-center" > < input type = "submit" value = "Create" class = "btn btn-primary" /> </ div > </ div > </ div > </ form > </ div > @section Scripts { @{await Html.RenderPartialAsync("_ValidationScriptsPartial");} < script type = "text/javascript" > $('#Content').summernote({ height: 300, // set editor height minHeight: null, // set minimum height of editor maxHeight: null, // set maximum height of editor focus: true, toolbar: [ ['style', ['style']], ['Insert', ['picture', 'link', 'video', 'table', 'hr']], ['font', ['bold', 'underline', 'clear']], ['fontname', ['fontname']], ['color', ['color']], ['para', ['ul', 'ol', 'paragraph']], ['table', ['table']], ['view', ['fullscreen', 'codeview', 'help']] ] }); </ script > } |
Dashboard.cshtml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 | @using Blog.BAL.Helpers @model PaginatedList< Blog.BAL.Models.PostViewModel > @{ ViewData["Title"] = "Dashboard"; } < div class = "container" > < br /> < p > < a asp-action = "Create" >Create New</ a > </ p > @if (Model.Count > 0) { < form asp-action = "Dashboard" method = "get" > < div class = "form-actions no-color" > < table style = "width:100%;" > < tr > < td style = "width:5%" >Filter:</ td > < td style = "width:30%" > < input type = "text" name = "SearchString" class = "form-control" value = "@ViewData[" CurrentFilter"]" /> </ td > < td > < input type = "submit" value = "Search" class = "btn btn-outline-info" /> | < a asp-action = "Dashboard" >Back to Full List</ a > </ td > </ tr > </ table > </ div > </ form > < div class = "table-responsive mb-1" > < table class = "table table-hover table-bordered m-0" > < thead > < tr > < th style = "white-space: nowrap;" > < a asp-action = "Dashboard" asp-route-sortOrder = "@ViewData[" TitleSortParm"]" asp-route-currentFilter = "@ViewData[" CurrentFilter"]"> Title</ a > </ th > < th style = "white-space: nowrap;" > < a asp-action = "Dashboard" asp-route-sortOrder = "@ViewData[" CategorySortParm"]" asp-route-currentFilter = "@ViewData[" CurrentFilter"]">Category</ a > </ th > < th style = "white-space: nowrap;" > < a asp-action = "Dashboard" asp-route-sortOrder = "@ViewData[" SeriesSortParm"]" asp-route-currentFilter = "@ViewData[" CurrentFilter"]">Series</ a > </ th > < th style = "white-space: nowrap;" > < a asp-action = "Dashboard" asp-route-sortOrder = "@ViewData[" AuthorNameSortParm"]" asp-route-currentFilter = "@ViewData[" CurrentFilter"]">Author Name</ a > </ th > < th style = "white-space: nowrap;" > < a asp-action = "Dashboard" asp-route-sortOrder = "@ViewData[" DateSortParm"]" asp-route-currentFilter = "@ViewData[" CurrentFilter"]">Published Date</ a > </ th > < th ></ th > </ tr > </ thead > < tbody > @foreach (var item in Model) { < tr > < td class = "col-3" style = "width: 25%; white-space: nowrap;" > @Html.DisplayFor(modelItem => item.Title) </ td > < td class = "col-2" style = "width: 20%; white-space: nowrap;" > @Html.DisplayFor(modelItem => item.Category) </ td > < td class = "col-2" style = "width: 20%; white-space: nowrap;" > @Html.DisplayFor(modelItem => item.Series) </ td > < td class = "col-2" style = "width: 10%; white-space: nowrap;" > @Html.DisplayFor(modelItem => item.AuthorName) </ td > < td class = "col-2" style = "width: 10%; white-space: nowrap;" > @item.PublishedDate.ToString("dd/MM/yyyy") </ td > < td class = "col-1" style = "width: 15%; white-space: nowrap;" > < a asp-controller = "Blog" asp-action = "Edit" asp-route-url = "@item.Content" class = "edit" title = "Edit" aria-expanded = "false" > < i class = "fas fa-edit" ></ i > </ a > | < a asp-controller = "Home" asp-action = "Index" asp-route-url = "@item.Content" class = "cancel" title = "View" aria-expanded = "false" > < i class = "fas fa-eye" ></ i > </ a > | @if (item.IsActive) { < a asp-controller = "Blog" asp-action = "Archive" asp-route-url = "@item.Content" class = "delete" title = "Archive" aria-expanded = "false" > < i class = "fas fa-trash" ></ i > </ a > } else { < a asp-controller = "Blog" asp-action = "Archive" asp-route-url = "@item.Content" class = "delete" title = "Restore" aria-expanded = "false" > < i class = "fas fa-trash-restore" ></ i > </ a > } </ td > </ tr > } </ tbody > </ table > </ div > var prevDisabled = !Model.HasPreviousPage ? "d-none" : ""; var nextDisabled = !Model.HasNextPage ? "d-none" : ""; < a asp-action = "Dashboard" asp-route-sortOrder = "@ViewData[" CurrentSort"]" asp-route-pageNumber = "@(Model.PageIndex - 1)" asp-route-currentFilter = "@ViewData[" CurrentFilter"]" class = "btn btn-outline-dark @prevDisabled" > Previous </ a > < a asp-action = "Dashboard" asp-route-sortOrder = "@ViewData[" CurrentSort"]" asp-route-pageNumber = "@(Model.PageIndex + 1)" asp-route-currentFilter = "@ViewData[" CurrentFilter"]" class = "btn btn-outline-dark @nextDisabled" > Next </ a > < br /> < br /> } </ div > @section Scripts { @{await Html.RenderPartialAsync("_ValidationScriptsPartial");} } |
Edit.cshtml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 | @model Blog.BAL.Models.PostViewModel @{ ViewData["Title"] = "Edit Post"; } < div class = "container" > < br /> < div class = "pb-3" > < a asp-action = "Dashboard" >Back to Dashboard</ a > </ div > < form asp-action = "Edit" enctype = "multipart/form-data" > < div class = "row" > < div class = "col-4" > < div class = "form-group" > < label asp-for = "Title" class = "control-label" ></ label > < input asp-for = "Title" class = "form-control" /> < input type = "hidden" asp-for = "ID" /> < input type = "hidden" asp-for = "Preview" /> < input type = "hidden" asp-for = "ViewCount" /> < input type = "hidden" asp-for = "LikeCount" /> < input type = "hidden" asp-for = "IsActive" value = "true" /> < span asp-validation-for = "Title" class = "text-danger" ></ span > </ div > </ div > < div class = "col-4" > < label asp-for = "AuthorName" class = "control-label" ></ label > < input asp-for = "AuthorName" class = "form-control" /> < span asp-validation-for = "AuthorName" class = "text-danger" ></ span > </ div > < div class = "col-4" > < div class = "form-group" > < label asp-for = "FormFile" class = "control-label" ></ label > < div class = "custom-file mb-3" > < input asp-for = "FormFile" type = "file" id = "filePostThumbnail" > </ div > < span asp-validation-for = "FormFile" class = "text-danger" ></ span > </ div > </ div > </ div > < div class = "row" > < div class = "col-4" > < label asp-for = "Category" class = "control-label" ></ label > < input asp-for = "Category" class = "form-control" /> < span asp-validation-for = "Category" class = "text-danger" ></ span > </ div > < div class = "col-4" > < label asp-for = "Series" class = "control-label" ></ label > < input asp-for = "Series" class = "form-control" /> < span asp-validation-for = "Series" class = "text-danger" ></ span > </ div > < div class = "col-4" > < label asp-for = "PublishedDate" class = "control-label" ></ label > < input asp-for = "PublishedDate" class = "form-control" /> < span asp-validation-for = "PublishedDate" class = "text-danger" ></ span > </ div > </ div > < div class = "row pt-3" > < div class = "col-12" > < label asp-for = "Content" class = "control-label" ></ label > < textarea asp-for = "Content" class = "form-control" rows = "5" ></ textarea > < span asp-validation-for = "Content" class = "text-danger" ></ span > </ div > </ div > < div class = "row pt-3" > < div class = "col-12" > < label asp-for = "TagsString" class = "control-label" ></ label > < input asp-for = "TagsString" class = "form-control" /> < span asp-validation-for = "TagsString" class = "text-danger" ></ span > </ div > </ div > < div class = "row pt-2" > < div class = "col-12" > < div class = "form-group text-center" > < input type = "submit" value = "Edit" class = "btn btn-large btn-primary" /> </ div > </ div > </ div > </ form > </ div > @section Scripts { @{await Html.RenderPartialAsync("_ValidationScriptsPartial");} < script type = "text/javascript" > $('#Content').summernote({ height: 300, // set editor height minHeight: null, // set minimum height of editor maxHeight: null, // set maximum height of editor focus: true, toolbar: [ ['style', ['style']], ['Insert', ['picture', 'link', 'video', 'table', 'hr']], ['font', ['bold', 'underline', 'clear']], ['fontname', ['fontname']], ['color', ['color']], ['para', ['ul', 'ol', 'paragraph']], ['table', ['table']], ['view', ['fullscreen', 'codeview', 'help']] ] }); </ script > } |
Index.cshtml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 369 370 371 372 373 374 375 | @model BlogViewModel @using Blog.BAL.Helpers @{ ViewData["Title"] = "Blog"; } < div class = "container" > < br /> < div > @if (MySession.Get(MyAppContext.Current, "__MyBlogCookie__") != null) { < p > < a asp-action = "Index" >Blog</ a > | < a asp-action = "Dashboard" >Go to Dashboard</ a > </ p > } @if (ViewData["tagFilter"] != null) { < span >Tag: < a asp-action = "Index" asp-controller = "Blog" asp-route-tagFilter = "@ViewData[" tagFilter"]">@ViewData["tagFilter"]</ a ></ span > } else if (ViewData["catFilter"] != null) { < span >Category: < a asp-action = "Index" asp-controller = "Blog" asp-route-catFilter = "@ViewData[" catFilter"]">@ViewData["catFilter"]</ a ></ span > } </ div > <!--========================================== BLOG PAGE ===========================================--> < div class = "container blog-page pl-0 pr-0" > < div class = "row" > <!-- Blog Posts --> < div class = "col-lg-8 col-12" > @if (Model.PagedBlog.Count() > 0) { <!-- Blog Post Wrapper --> < div class = "blog-posts-wrapper" > @foreach (var post in Model.PagedBlog) { < div class = "card blog" data-aos = "fade-up" data-aos-duration = "500" data-aos-delay = "100" > <!-- Blog image here --> < a asp-action = "Index" asp-controller = "Home" asp-route-url = "@post.Content" > < img class = "img-responsive" src = "/Uploads/BlogImages/@post.Thumbnail" > </ a > < div class = "blog-content" > <!-- Edit title and info here --> < div class = "blog-title" > < a asp-action = "Index" asp-controller = "Home" asp-route-url = "@post.Content" > < h1 class = "text-capitalize" >@post.Title</ h1 > </ a > < div class = "row" > <!--<div class="col-12 col-lg-4 text-left"> <ul>--> @*< li >< i class = "fa fa-user text-warning" ></ i >@post.AuthorName</ li >*@ <!--</ul> </div>--> < div class = "col-12 text-right" > < ul > < li >< i class = "fa fa-calendar text-info" ></ i >@post.PublishedDate.ToString("MMM dd, yyyy")</ li > < li >< i class = "fa fa-heart text-danger" ></ i >@post.LikeCount</ li > < li >< i class = "fa fa-eye text-success" ></ i >@post.ViewCount</ li > </ ul > </ div > </ div > </ div > <!-- some line of post --> < p class = "text-left" > @post.Preview ... </ p > </ div > </ div > <!-- In-feed Ads --> <!-- In-feed Ads --> < div class = "card blog" data-aos = "fade-up" data-aos-duration = "500" data-aos-delay = "100" > <!-- Blog Native Square Resp. Ads --> < ins class = "adsbygoogle" style = "display:block;" data-ad-client = "ca-pub-5799253124543293" data-ad-slot = "2768814167" data-ad-format = "auto" data-full-width-responsive = "true" ></ ins > < script > (adsbygoogle = window.adsbygoogle || []).push({}); </ script > </ div > } </ div > var prevDisabled = !Model.PagedBlog.HasPreviousPage ? "d-none" : ""; var nextDisabled = !Model.PagedBlog.HasNextPage ? "d-none" : ""; < div class = "pb-4" > < a asp-action = "Index" asp-route-tagFilter = "@ViewData[" tagFilter"]" asp-route-catFilter = "@ViewData[" catFilter"]" asp-route-pageNumber = "@(Model.PagedBlog.PageIndex - 1)" asp-route-currentFilter = "@ViewData[" CurrentFilter"]" class = "btn btn-outline-dark @prevDisabled" > Previous </ a > < a asp-action = "Index" asp-route-tagFilter = "@ViewData[" tagFilter"]" asp-route-catFilter = "@ViewData[" catFilter"]" asp-route-pageNumber = "@(Model.PagedBlog.PageIndex + 1)" asp-route-currentFilter = "@ViewData[" CurrentFilter"]" class = "btn btn-outline-dark @nextDisabled" > Next </ a > </ div > } else { < p >Oops! Can't find any post here!</ p > <!-- In-feed Ads --> < div class = "card blog" data-aos = "fade-up" data-aos-duration = "500" data-aos-delay = "100" > < ins class = "adsbygoogle" style = "display:block;" data-ad-format = "fluid" data-ad-layout-key = "-dw+6n-2f-dh+x9" data-ad-client = "ca-pub-5799253124543293" data-ad-slot = "5304667539" ></ ins > < script > (adsbygoogle = window.adsbygoogle || []).push({}); </ script > </ div > } </ div > <!-- ./Blog Posts --> <!-- Sidebar --> < div class = "col-lg-4 col-12" > < div class = "sidebar" > <!-- Search --> < div class = "search card" > < form asp-action = "Index" method = "get" > < div class = "input-group" > < input id = "search" name = "SearchString" type = "text" class = "validate" placeholder = "Search Here" value = "@ViewData[" CurrentFilter"]"> < span class = "input-group-btn" > < button name = "submit" type = "submit" class = "btn btn-outline-dark" data-aos = "zoom-in" data-aos-duration = "10" data-aos-delay = "10" > < i class = "fas fa-search" ></ i > </ button > </ span > </ div > </ form > </ div > <!-- ./Search --> <!-- Normal Native Sqaure Ads --> < div class = "card sidebar-post" > <!-- Blog Native Square Resp. Ads --> < ins class = "adsbygoogle" style = "display:block;" data-ad-client = "ca-pub-5799253124543293" data-ad-slot = "2768814167" data-ad-format = "auto" data-full-width-responsive = "true" ></ ins > < script > (adsbygoogle = window.adsbygoogle || []).push({}); </ script > </ div > <!-- Category --> < h2 class = "title text-capitalize" >Categories</ h2 > < ul class = "category" > @foreach (var cat in Model.Categories) { if (cat != "All") { < li >< a asp-action = "Index" asp-route-catFilter = "@cat" >@cat</ a ></ li > } else { < li >< a asp-action = "Index" asp-route-catFilter = "" >@cat</ a ></ li > } } </ ul > <!-- ./Category --> <!-- Normal Native Sqaure Ads --> < div class = "card sidebar-post" > <!-- Blog Native Square Resp. Ads --> < ins class = "adsbygoogle" style = "display:block;" data-ad-client = "ca-pub-5799253124543293" data-ad-slot = "2768814167" data-ad-format = "auto" data-full-width-responsive = "true" ></ ins > < script > (adsbygoogle = window.adsbygoogle || []).push({}); </ script > </ div > <!-- Popular Posts --> < h2 class = "title text-capitalize" >Popular Posts</ h2 > < div class = "card sidebar-post" > @foreach (var post in Model.PopularPosts) { <!-- Popular 1 --> < div class = "row" > <!-- Sidebar post image --> < div class = "col-3" > < div class = "center-block mx-auto" style = "width:50px" > < a asp-action = "Index" asp-controller = "Home" asp-route-url = "@post.Content" > < img class = "img-circle center-block" style = "width: 50px; height:50px;" src = "/Uploads/BlogImages/@post.Thumbnail" alt = "" > </ a > </ div > </ div > <!-- Post Headline with link --> < div class = "col-9" > < a asp-action = "Index" asp-controller = "Home" asp-route-url = "@post.Content" >< p class = "text-capitalize text-underline" >@post.Title</ p ></ a > < p >@post.PublishedDate.ToShortDateString()</ p > </ div > </ div > } </ div > <!-- Normal Native Sqaure Ads --> < div class = "card sidebar-post" > <!-- Blog Native Square Resp. Ads --> < ins class = "adsbygoogle" style = "display:block;" data-ad-client = "ca-pub-5799253124543293" data-ad-slot = "2768814167" data-ad-format = "auto" data-full-width-responsive = "true" ></ ins > < script > (adsbygoogle = window.adsbygoogle || []).push({}); </ script > </ div > <!-- ./Recent Posts --> <!-- Popular Posts --> < h2 class = "title text-capitalize" >Favorite Posts</ h2 > < div class = "card sidebar-post" > @foreach (var post in Model.FavoritePosts) { <!-- Popular 1 --> < div class = "row" > <!-- Sidebar post image --> < div class = "col-3" > < div class = "center-block mx-auto" style = "width:50px" > < a asp-action = "Index" asp-controller = "Home" asp-route-url = "@post.Content" > < img class = "img-circle center-block" style = "width: 50px; height:50px;" src = "/Uploads/BlogImages/@post.Thumbnail" alt = "" > </ a > </ div > </ div > <!-- Post Headline with link --> < div class = "col-9" > < a asp-action = "Index" asp-controller = "Home" asp-route-url = "@post.Content" >< p class = "text-capitalize text-underline" >@post.Title</ p ></ a > < p >@post.PublishedDate.ToShortDateString()</ p > </ div > </ div > } </ div > <!-- ./Popular Posts --> <!-- Normal Native Sqaure Ads --> < div class = "card sidebar-post" > <!-- Blog Native Square Resp. Ads --> < ins class = "adsbygoogle" style = "display:block;" data-ad-client = "ca-pub-5799253124543293" data-ad-slot = "2768814167" data-ad-format = "auto" data-full-width-responsive = "true" ></ ins > < script > (adsbygoogle = window.adsbygoogle || []).push({}); </ script > </ div > <!-- About the Author --> < h2 class = "title text-capitalize" >About The Author</ h2 > < div class = "card sidebar-post" > < div id = "profile" class = "center-block mx-auto" > < img src = "/Uploads/UserImages/@Model.AboutMe.ProfileImage" style = "width:150px; height:150px;" class = "img-circle center-block author-profile" alt = "" /> </ div > < p class = "text-center author-info" > @Model.AboutMe.Summary </ p > < div class = "row text-center mx-auto" > < div class = "text-center text-dark" > < a asp-action = "Index" asp-controller = "AboutMe" target = "_blank" class = "text-uppercase visit-profile btn" > Visit My Profile </ a > </ div > </ div > </ div > <!-- ./About the Author --> <!-- Normal Native Sqaure Ads --> < div class = "card sidebar-post" > <!-- Blog Native Square Resp. Ads --> < ins class = "adsbygoogle" style = "display:block;" data-ad-client = "ca-pub-5799253124543293" data-ad-slot = "2768814167" data-ad-format = "auto" data-full-width-responsive = "true" ></ ins > < script > (adsbygoogle = window.adsbygoogle || []).push({}); </ script > </ div > <!-- Follow Me --> < h2 class = "title text-capitalize" >Follow</ h2 > < div class = "card sidebar-post" id = "default-width" > < ul class = "text-center list-inline h1" > < li class = "list-inline-item" >< a href = "https://www.linkedin.com/in/nguyenhm/" target = "_blank" >< i class = "fab fa-linkedin-in" ></ i ></ a ></ li > < li class = "list-inline-item" >< a href = "https://github.com/lucas-ngminh" target = "_blank" >< i class = "fab fa-github" ></ i ></ a ></ li > < li class = "list-inline-item" >< a href = "https://www.youtube.com/channel/UCMc-1Gb8xFkyUIlwqpPmS1Q" target = "_blank" >< i class = "fab fa-youtube" ></ i ></ a ></ li > < li class = "list-inline-item" >< a href = "https://www.facebook.com/lucasology" target = "_blank" >< i class = "fab fa-facebook" ></ i ></ a ></ li > < li class = "list-inline-item" >< a href = "https://www.instagram.com/lucas.ology" target = "_blank" >< i class = "fab fa-instagram" ></ i ></ a ></ li > </ ul > </ div > <!-- Vertical Ad --> < div class = "fixed-position-start" ></ div > < div class = "card sidebar-post vertical-fixed-ad" > <!-- Blog Vertical Display Ads --> < ins class = "adsbygoogle" style = "display:block;" data-ad-client = "ca-pub-5799253124543293" data-ad-slot = "1725349324" data-ad-format = "auto" data-full-width-responsive = "true" ></ ins > < script > (adsbygoogle = window.adsbygoogle || []).push({}); </ script > < div class = "d-xl-block d-lg-none" > <!-- Blog Vertical Display Ads --> < ins class = "adsbygoogle" style = "display:block;" data-ad-client = "ca-pub-5799253124543293" data-ad-slot = "1725349324" data-ad-format = "auto" data-full-width-responsive = "true" ></ ins > < script > (adsbygoogle = window.adsbygoogle || []).push({}); </ script > </ div > </ div > </ div > </ div > </ div > <!-- ./Sidebar --> </ div > </ div > @section Scripts { @{await Html.RenderPartialAsync("_ValidationScriptsPartial");} } |
c. In Error folder:
Index.cshtml
1 2 3 4 5 6 7 | < div class = "text-center welcome" style = "background:@ViewData[" bgColor"]; color:@ViewData["color"]; background-image:url('/images/assets/@ViewData["error"]');"> < div class = "container-fluid" > < h1 class = "display-4 pt-2" >@ViewData["message"]</ h1 > < p >< a href = "javascript:void(0);" onclick = "history.go(-1);" >Go Back</ a ></ p > </ div > </ div > |
d. In Home folder:
Contact.cshtml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 | @{ ViewData["Title"] = "Privacy Policy"; } < div class = "container" > <!--========================================== CONTACT ===========================================--> < section id = "contact" class = "section" > < h1 class = "text-uppercase text-left" >Contact</ h1 > < div class = "container" > < div class = "row" > <!-- Contact Info --> < div class = "col-12" > < div class = "info card" > < br />< br />< br /> <!-- Apply your own info here --> < ul class = "text-center center-block" data-aos = "fade" data-aos-delay = "200" data-aos-offset = "0" > < li > < i class = "fa fa-envelope center-block" ></ i >< br /> </ li > < li > < i class = "fa fa-globe center-block" ></ i >< br /> < a class = "text-white" asp-action = "Index" asp-controller = "Home" >lucasology.com</ a > </ li > < li > < i class = "fa fa-location-arrow center-block" ></ i >< br /> < span >Brooklyn, NY - 11204</ span > </ li > </ ul > </ div > </ div > <!-- ./Contact Info --> <!-- Contact Form --> <!--<div class="col col-md-7 col-sm-6 col-xs-12"> <div class="contact card"> <form id="contact-form" name="contact-form" role="form"> <div class="input-field"> <input id="name" name="name" type="text" class="validate" required> <label for="name">Name</label> </div> <div class="input-field"> <input id="subject" name="subject" type="text" class="validate" required> <label for="subject">Subject</label> </div> <div class="input-field"> <input id="email" name="email" type="email" class="validate" required> <label for="email">Email</label> </div> <div class="input-field"> <textarea id="textarea" name="message" class="materialize-textarea" required></textarea> <label for="textarea">Massage</label> </div> <div class="row"> <div class="col col-md-12 col-sm-12 col-xs-12 text-center"> <button type="submit" id="submit" name="submit-data" value="Submit" class="btn waves-effect waves-light btn-primary custom-btn">--> <!-- Button value here --> <!--<i class="ion-ios-paperplane"></i>Send </button> </div>--> <!-- Message Sent and Error info --> <!--<div id="snackbar">Your Message is sent</div> <div id="fail-snackbar">Something is going wrong, Please try again</div> </div> </form> </div> </div>--> <!-- ./Contact Form --> </ div > <!-- Location MAP --> <!--<div class="row"> <div class="col col-md-12 col-sm-12 col-xs-12"> <div id="google-map" data-aos="zoom-in" data-aos-delay="0" data-aos-offset="0"> <div id="map-container"></div> <div id="cd-zoom-in" class="custom-btn"><i class="material-icons text-center">add</i></div> <div id="cd-zoom-out" class="custom-btn"><i class="material-icons text-center">remove</i></div>--> <!-- Your address here --> <!--<address class="text-center">25/A New Eskaton Road, Maghbazar, Dhaka 1000, Bangladesh</address> </div> </div> </div>--> </ div > </ section > </ div > |
Index.cshtml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 369 370 371 372 373 374 375 376 377 378 379 380 381 382 383 384 385 386 387 388 389 390 391 392 393 394 395 396 397 398 399 400 401 402 403 404 405 406 407 408 409 410 411 412 413 414 415 416 417 418 419 420 421 422 423 424 425 426 427 428 429 430 431 432 433 434 435 436 437 438 439 440 441 442 443 444 445 446 447 448 449 450 451 452 453 | @model BlogViewModel @{ ViewData["Title"] = "Home"; } @if (Model == null) { < div class = "text-center welcome" style = "background:@ViewData[" bgColor"]; color:@ViewData["color"]; background-image:url('./images/assets/@ViewData["welcome"]');"> < div class = "container-fluid" > < h1 class = "display-4 pt-2" >Welcome</ h1 > < p >< a style = "color:@ViewData[" color"]" asp-action = "Index" asp-controller = "AboutMe" >About Me?</ a ></ p > </ div > </ div > } else { < div class = "container" > < br /> < div > < a asp-action = "Index" asp-controller = "Blog" >Blog</ a > / < a asp-action = "Index" asp-controller = "Blog" asp-route-catFilter = "@Model.PagedBlog[0].Category" >@Model.PagedBlog[0].Category</ a > </ div > <!--========================================== BLOG PAGE ===========================================--> < div class = "container blog-page pl-0 pr-0" > < div class = "row" > <!-- Blog Posts --> < div class = "col-lg-8 col-12" > @if (Model.PagedBlog.Count() > 0) { <!-- Blog Post Wrapper --> < div class = "blog-posts-wrapper" > <!-- Single Posts --> < div > <!-- Blog Post Wrapper --> < div class = "blog-posts-wrapper" > <!-- Full post --> < div class = "card blog full-post" > <!-- Blog image here --> < img class = "img-responsive" src = "/Uploads/BlogImages/@Model.PagedBlog[0].Thumbnail" > < div class = "blog-content" > <!-- Edit title and info here --> < div class = "blog-title post-title" > < h5 class = "text-capitalize" >@Model.PagedBlog[0].Title</ h5 > < div class = "row" > <!--<div class="col-12 col-lg-4 text-left"> <ul>--> @*< li >< i class = "fa fa-user text-warning" ></ i >@Model.PagedBlog[0].AuthorName</ li >*@ <!--</ul> </div>--> < div class = "col-12 text-right" > < ul > < li >< i class = "fa fa-calendar text-info" ></ i >@Model.PagedBlog[0].PublishedDate.ToString("MMM dd, yyyy")</ li > < li >< i class = "fa fa-heart text-danger" ></ i >@Model.PagedBlog[0].LikeCount</ li > < li >< i class = "fa fa-eye text-success" ></ i >@Model.PagedBlog[0].ViewCount</ li > </ ul > </ div > </ div > </ div > < div class = "content-menu" > @if(Model.PagedBlog[0].Contents.Count() > 0) { < h2 >Contents</ h2 > < ul > @for (int i = 0; i < Model.PagedBlog [0].Contents.Count(); i++) { if (@Model.PagedBlog[0].Contents[i].StartsWith("Step")) { <li class = "pl-4" > < a href = "#section_@i" > @Model.PagedBlog[0].Contents[i] </ a > </ li > } else { < li > < a href = "#section_@i" > @Model.PagedBlog[0].Contents[i] </ a > </ li > } } </ ul > } </ div > < div class = "postcontent" > @Html.Raw(Model.PagedBlog[0].Content) </ div > < div class = "pt-1" > @if (Model.PagedBlog[0].SeriesPosts.Count() > 0) { < h2 >Read more in this series:</ h2 > < ul > @foreach (var cat in Model.PagedBlog[0].SeriesPosts.Select(s => s.Category).Distinct().ToList()) { < li >@cat: < ol > @foreach (var post in Model.PagedBlog[0].SeriesPosts.Where(p => p.Category == cat).ToList()) { if (post.ID == Model.PagedBlog[0].ID) { < li > < span >< b >You are reading:</ b > </ span >@post.Title </ li > } else { < li > < a asp-action = "Index" asp-controller = "Home" asp-route-url = "@post.Content" > @post.Title </ a > </ li > } } </ ol > </ li > } </ ul > } </ div > </ div > < hr > @if (Model.PagedBlog[0].Tags.Count() > 0) { < div class = "tags" > < i class = "fa fa-tags" ></ i > < span class = "text-info" > @foreach (var t in Model.PagedBlog[0].Tags) { < a asp-action = "Index" asp-controller = "Blog" asp-route-tagFilter = "@t" > < span class = "badge badge-primary" >@t</ span > </ a > } </ span > </ div > } </ div > <!-- ./Full post --> </ div > </ div > <!-- ./Single Posts --> </ div > < div class = "card blog" > < div class = "fb-comments" data-href = "@ViewBag.PostUrl" data-numposts = "5" data-width = "100%" ></ div > </ div > if (Model.PagedBlog.Count() > 1) { @for (int i = 1; i < Model.PagedBlog.Count (); i++) { <div class = "card blog" > <!-- Blog image here --> < a asp-action = "Index" asp-controller = "Home" asp-route-url = "@Model.PagedBlog[i].Content" > < img class = "img-responsive" src = "/Uploads/BlogImages/@Model.PagedBlog[i].Thumbnail" > </ a > < div class = "blog-content" > <!-- Edit title and info here --> < div class = "blog-title" > < a asp-action = "Index" asp-controller = "Home" asp-route-url = "@Model.PagedBlog[i].Content" > < h1 class = "text-capitalize" >@Model.PagedBlog[i].Title</ h1 > </ a > < div class = "row" > <!--<div class="col-12 col-lg-4 text-left"> <ul>--> @*< li >< i class = "fa fa-user text-warning" ></ i >@Model.PagedBlog[i].AuthorName</ li >*@ <!--</ul> </div>--> < div class = "col-12 text-right" > < ul > < li >< i class = "fa fa-calendar text-info" ></ i >@Model.PagedBlog[i].PublishedDate.ToString("MMM dd, yyyy")</ li > < li >< i class = "fa fa-heart text-danger" ></ i >@Model.PagedBlog[i].LikeCount</ li > < li >< i class = "fa fa-eye text-success" ></ i >@Model.PagedBlog[i].ViewCount</ li > </ ul > </ div > </ div > </ div > <!-- some line of post --> < p class = "text-left" > @Model.PagedBlog[i].Preview ... </ p > </ div > </ div > <!-- In-feed Ads --> < div class = "card blog" > <!-- Blog Native Square Resp. Ads --> < ins class = "adsbygoogle" style = "display:block;" data-ad-client = "ca-pub-5799253124543293" data-ad-slot = "2768814167" data-ad-format = "auto" data-full-width-responsive = "true" ></ ins > < script > (adsbygoogle = window.adsbygoogle || []).push({}); </ script > </ div > } } } </ div > <!-- ./Blog Posts --> <!-- Sidebar --> < div class = "col-lg-4 col-12" > < div class = "sidebar" > <!-- Search --> < div class = "search card" > < form asp-action = "Index" asp-controller = "Blog" method = "get" > < div class = "input-group" > < input id = "search" name = "SearchString" type = "text" class = "validate" placeholder = "Search Here" value = "@ViewData[" CurrentFilter"]"> < span class = "input-group-btn" > < button name = "submit" type = "submit" class = "btn btn-outline-dark" data-aos = "zoom-in" data-aos-duration = "10" data-aos-delay = "10" > < i class = "fas fa-search" ></ i > </ button > </ span > </ div > </ form > </ div > <!-- Normal Native Sqaure Ads --> < div class = "card sidebar-post" > <!-- Blog Native Square Resp. Ads --> < ins class = "adsbygoogle" style = "display:block;" data-ad-client = "ca-pub-5799253124543293" data-ad-slot = "2768814167" data-ad-format = "auto" data-full-width-responsive = "true" ></ ins > < script > (adsbygoogle = window.adsbygoogle || []).push({}); </ script > </ div > <!-- ./Search --> <!-- Category --> < h2 class = "title text-capitalize" >Categories</ h2 > < ul class = "category" > @foreach (var cat in Model.Categories) { if (cat != "All") { < li >< a asp-action = "Index" asp-controller = "Blog" asp-route-catFilter = "@cat" >@cat</ a ></ li > } else { < li >< a asp-action = "Index" asp-controller = "Blog" asp-route-catFilter = "" >@cat</ a ></ li > } } </ ul > <!-- ./Category --> <!-- Normal Native Sqaure Ads --> < div class = "card sidebar-post" > <!-- Blog Native Square Resp. Ads --> < ins class = "adsbygoogle" style = "display:block;" data-ad-client = "ca-pub-5799253124543293" data-ad-slot = "2768814167" data-ad-format = "auto" data-full-width-responsive = "true" ></ ins > < script > (adsbygoogle = window.adsbygoogle || []).push({}); </ script > </ div > <!-- Popular Posts --> < h2 class = "title text-capitalize" >Popular Posts</ h2 > < div class = "card sidebar-post" > @foreach (var post in Model.PopularPosts) { <!-- Popular 1 --> < div class = "row" > <!-- Sidebar post image --> < div class = "col-3" > < div class = "center-block mx-auto" style = "width:50px" > < a asp-action = "Index" asp-controller = "Home" asp-route-url = "@post.Content" > < img class = "img-circle center-block" style = "width: 50px; height:50px;" src = "/Uploads/BlogImages/@post.Thumbnail" alt = "" > </ a > </ div > </ div > <!-- Post Headline with link --> < div class = "col-9" > < a asp-action = "Index" asp-controller = "Home" asp-route-url = "@post.Content" >< p class = "text-capitalize text-underline" >@post.Title</ p ></ a > < p >@post.PublishedDate.ToShortDateString()</ p > </ div > </ div > } </ div > <!-- Normal Native Sqaure Ads --> < div class = "card sidebar-post" > <!-- Blog Native Square Resp. Ads --> < ins class = "adsbygoogle" style = "display:block;" data-ad-client = "ca-pub-5799253124543293" data-ad-slot = "2768814167" data-ad-format = "auto" data-full-width-responsive = "true" ></ ins > < script > (adsbygoogle = window.adsbygoogle || []).push({}); </ script > </ div > <!-- ./Recent Posts --> <!-- Popular Posts --> < h2 class = "title text-capitalize" >Favorite Posts</ h2 > < div class = "card sidebar-post" > @foreach (var post in Model.FavoritePosts) { <!-- Popular 1 --> < div class = "row" > <!-- Sidebar post image --> < div class = "col-3" > < div class = "center-block mx-auto" style = "width:50px" > < a asp-action = "Index" asp-controller = "Home" asp-route-url = "@post.Content" > < img class = "img-circle center-block" style = "width: 50px; height:50px;" src = "/Uploads/BlogImages/@post.Thumbnail" alt = "" > </ a > </ div > </ div > <!-- Post Headline with link --> < div class = "col-9" > < a asp-action = "Index" asp-controller = "Home" asp-route-url = "@post.Content" >< p class = "text-capitalize text-underline" >@post.Title</ p ></ a > < p >@post.PublishedDate.ToShortDateString()</ p > </ div > </ div > } </ div > <!-- Normal Native Sqaure Ads --> < div class = "card sidebar-post" > <!-- Blog Native Square Resp. Ads --> < ins class = "adsbygoogle" style = "display:block;" data-ad-client = "ca-pub-5799253124543293" data-ad-slot = "2768814167" data-ad-format = "auto" data-full-width-responsive = "true" ></ ins > < script > (adsbygoogle = window.adsbygoogle || []).push({}); </ script > </ div > <!-- ./Popular Posts --> <!-- About the Author --> < h2 class = "title text-capitalize" >About The Author</ h2 > < div class = "card sidebar-post" > < div id = "profile" class = "center-block mx-auto" > < img src = "/Uploads/UserImages/@Model.AboutMe.ProfileImage" style = "width:150px; height:150px;" class = "img-circle center-block author-profile" alt = "" /> </ div > < p class = "text-center author-info" > @Model.AboutMe.Summary </ p > < div class = "row text-center mx-auto" > < div class = "text-center text-dark" > < a asp-action = "Index" asp-controller = "AboutMe" target = "_blank" class = "text-uppercase visit-profile btn" > Visit My Profile </ a > </ div > </ div > </ div > <!-- Normal Native Sqaure Ads --> < div class = "card sidebar-post" > <!-- Blog Native Square Resp. Ads --> < ins class = "adsbygoogle" style = "display:block;" data-ad-client = "ca-pub-5799253124543293" data-ad-slot = "2768814167" data-ad-format = "auto" data-full-width-responsive = "true" ></ ins > < script > (adsbygoogle = window.adsbygoogle || []).push({}); </ script > </ div > <!-- ./About the Author --> <!-- Follow Me --> < h2 class = "title text-capitalize" >Follow</ h2 > < div class = "card sidebar-post" id = "default-width" > < ul class = "text-center list-inline h1" > < li class = "list-inline-item" >< a href = "https://www.linkedin.com/in/nguyenhm/" target = "_blank" >< i class = "fab fa-linkedin-in" ></ i ></ a ></ li > < li class = "list-inline-item" >< a href = "https://github.com/lucas-ngminh" target = "_blank" >< i class = "fab fa-github" ></ i ></ a ></ li > < li class = "list-inline-item" >< a href = "https://www.youtube.com/channel/UCMc-1Gb8xFkyUIlwqpPmS1Q" target = "_blank" >< i class = "fab fa-youtube" ></ i ></ a ></ li > < li class = "list-inline-item" >< a href = "https://www.facebook.com/lucasology" target = "_blank" >< i class = "fab fa-facebook" ></ i ></ a ></ li > < li class = "list-inline-item" >< a href = "https://www.instagram.com/lucas.ology" target = "_blank" >< i class = "fab fa-instagram" ></ i ></ a ></ li > </ ul > </ div > <!-- Vertical Ad --> < div class = "fixed-position-start" ></ div > < div class = "card sidebar-post vertical-fixed-ad" > <!-- Blog Vertical Display Ads --> < ins class = "adsbygoogle" style = "display:block;" data-ad-client = "ca-pub-5799253124543293" data-ad-slot = "1725349324" data-ad-format = "auto" data-full-width-responsive = "true" ></ ins > < script > (adsbygoogle = window.adsbygoogle || []).push({}); </ script > < div class = "d-xl-block d-lg-none" > <!-- Blog Vertical Display Ads --> < ins class = "adsbygoogle" style = "display:block;" data-ad-client = "ca-pub-5799253124543293" data-ad-slot = "1725349324" data-ad-format = "auto" data-full-width-responsive = "true" ></ ins > < script > (adsbygoogle = window.adsbygoogle || []).push({}); </ script > </ div > </ div > </ div > </ div > <!-- ./Sidebar --> </ div > </ div > </ div > } |
Login.cshtml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 | @model Blog.BAL.Models.LoginViewModel @{ ViewData["Title"] = "Privacy Policy"; } < div class = "container" > < section class = "section" > < div class = "container" > < div class = "row" > < div class = "col-12 col-lg-3" ></ div > <!-- Login Info --> < div class = "col-12 col-lg-6" > < div class = "card" > < form asp-controller = "Home" asp-action = "Login" asp-route-returnUrl = "@ViewBag.ReturnUrl" > < div class = "modal-header" > < h5 class = "modal-title" >Login</ h5 > </ div > < div class = "modal-body" > @if (ViewData.ModelState[""] != null && ViewData.ModelState[""].Errors.Count > 0) { < div class = "alert alert-danger" > < button type = "button" class = "close" data-dismiss = "alert" >×</ button > @Html.ValidationSummary(true, "Errors: ") </ div > } < input name = "IsValid" type = "hidden" value = "@ViewData.ModelState.IsValid.ToString()" /> < div class = "form-group" > < label asp-for = "Password" ></ label > < input type = "password" asp-for = "Password" class = "form-control" /> < span asp-validation-for = "Password" class = "text-danger" ></ span > </ div > < div class = "form-group" > < input type = "checkbox" id = "cbRememberMe" asp-for = "RememberMe" /> < label for = "cbRememberMe" >Remember Me</ label > </ div > </ div > < div class = "modal-footer" > < button type = "submit" class = "btn btn-primary" data-save = "modal" >Login</ button > </ div > </ form > </ div > </ div > < div class = "col-12 col-lg-3" ></ div > </ div > </ div > </ section > </ div > |
d. In Shared folder:
_Layout.cshtml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 | @using Blog.BAL.Helpers <!DOCTYPE html> < html > < head > < meta charset = "utf-8" /> < meta name = "viewport" content = "width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=no" /> < meta property = "fb:app_id" content = "426198148401369" /> < meta property = "fb:admins" content = "nguynminhoang" /> < meta property = "og:locale" content = "en_US" /> < meta property = "og:type" content = "article" /> < meta property = "og:site_name" content = "Lucasology" /> < meta property = "og:url" content = "@ViewBag.PostUrl" /> < meta property = "og:title" content = "@ViewBag.ArticleTitle" /> < meta property = "og:image" content = "@ViewBag.Thumbnail" /> < meta property = "og:description" content = "@ViewBag.Preview" /> < meta name = "twitter:card" content = "summary_large_image" /> < meta name = "twitter:title" content = "@ViewBag.ArticleTitle" /> < meta name = "twitter:image" content = "@ViewBag.TwitterThumbnail?v=1" /> < meta name = "twitter:description" content = "@ViewBag.Preview" /> < link rel = "icon" type = "image/png" sizes = "16x16" href = "~/favicon-16x16.png" /> < link rel = "icon" type = "image/png" sizes = "32x32" href = "~/favicon-32x32.png" /> < link rel = "icon" type = "image/png" sizes = "180x180" href = "~/apple-touch-icon.png" /> < link rel = "icon" type = "image/png" sizes = "192x192" href = "~/android-chrome-192x192" /> < link rel = "icon" type = "image/png" sizes = "512x512" href = "~/android-chrome-512x512" /> < link rel = "manifest" href = "~/site.webmanifest" /> @if (ViewBag.ArticleTitle == null) { < title >Lucas•o•lo•gy - @ViewData["Title"]</ title > } else { < title >Lucas•o•lo•gy - @ViewBag.ArticleTitle</ title > < link rel = "amphtml" href = "@ViewBag.PostUrl" > } @if (ViewBag.LdJsonObject != null) { < script type = "application/ld+json" > @Html.Raw(ViewBag.LdJsonObject) </ script > } <!-- Google Font --> < link rel = "stylesheet" href = "~/lib/bootstrap/dist/css/bootstrap.css" /> < link rel = "stylesheet" href = "~/css/site.css" /> < link href = "~/lib/font-awesome/css/all.css" rel = "stylesheet" /> < link href = "~/lib/summernote/summernote-bs4.css" rel = "stylesheet" /> < link href = "~/css/animate.css" rel = "stylesheet" /> < link href = "~/css/aos.min.css" rel = "stylesheet" /> < link href = "~/css/swiper.css" rel = "stylesheet" /> < link href = "~/lib/SyntaxHighlighter/styles/shCoreMidnight.css" rel = "stylesheet" /> <!-- Google Adsense --> < script data-ad-client = "ca-pub-5799253124543293" async src = "https://pagead2.googlesyndication.com/pagead/js/adsbygoogle.js" ></ script > </ head > < body > < div id = "fb-root" ></ div > < script async defer crossorigin = "anonymous" src = "https://connect.facebook.net/en_US/sdk.js#xfbml=1&version=v9.0&appId=426198148401369&autoLogAppEvents=1" nonce = "mgi53C8j" ></ script > < div id = "PlaceHolderHere" ></ div > < header > < nav class = "navbar navbar-expand-sm navbar-toggleable-sm navbar-dark bg-dark border-bottom box-shadow" > < div class = "container" > < a class = "navbar-brand text-white" asp-area = "" asp-controller = "Home" asp-action = "Index" >Lucas•o•lo•gy</ a > < button class = "navbar-toggler" type = "button" data-toggle = "collapse" data-target = ".navbar-collapse" aria-controls = "navbarSupportedContent" aria-expanded = "false" aria-label = "Toggle navigation" > < span class = "navbar-toggler-icon" ></ span > </ button > < div class = "navbar-collapse collapse d-sm-inline-flex flex-sm-row-reverse" > < ul class = "navbar-nav ml-auto" > < li class = "nav-item" > < a class = "nav-link text-white" asp-area = "" asp-controller = "Home" asp-action = "Index" >Home</ a > </ li > < li class = "nav-item" > < a class = "nav-link text-white" asp-area = "" asp-controller = "AboutMe" asp-action = "Index" >About Me</ a > </ li > @*< li class = "nav-item" > < a class = "nav-link text-white" asp-area = "" asp-controller = "Portfolio" asp-action = "Index" >Portfolio</ a > </ li >*@ < li class = "nav-item" > < a class = "nav-link text-white" asp-area = "" asp-controller = "Blog" asp-action = "Index" >Blog</ a > </ li > < li class = "nav-item" > < a class = "nav-link text-white" asp-area = "" asp-controller = "Home" asp-action = "Contact" >Contact</ a > </ li > </ ul > </ div > </ div > </ nav > </ header > < div class = "" > < main role = "main" > @RenderBody() </ main > </ div > < a href = "#header" id = "btp" class = "back-to-top btn-floating waves-effect waves-light btn-large custom-btn" > < i class = "fa fa-arrow-up" ></ i > </ a > < footer class = "border-top footer text-muted" > < div class = "container" > < div class = "float-right" > © @DateTime.Now.Year - Hoang - @if (MySession.Get(MyAppContext.Current, "__MyBlogCookie__") == null) { < button type = "button" class = "btn btn-link p-0 m-0 mb-1" data-toggle = "ajax-modal" data-target = "#login" data-url = "@Url.Action(" Login", "AboutMe")"> Sign In </ button > } else { < a href = "@Url.Action(" LogOut", "AboutMe", new { })">Sign Out</ a > } </ div > </ div > </ footer > < script src = "~/js/aos.min.js" ></ script > < script src = "~/lib/jquery/dist/jquery.min.js" ></ script > < script src = "~/lib/bootstrap/dist/js/bootstrap.bundle.min.js" ></ script > < script src = "~/lib/summernote/summernote-bs4.js" ></ script > < script src = "~/lib/SyntaxHighlighter/scripts/shCore.js" ></ script > < script src = "~/lib/SyntaxHighlighter/scripts/shAutoloader.js" ></ script > < script src = "~/lib/SyntaxHighlighter/scripts/shBrushAppleScript.js" ></ script > < script src = "~/lib/SyntaxHighlighter/scripts/shBrushAS3.js" ></ script > < script src = "~/lib/SyntaxHighlighter/scripts/shBrushBash.js" ></ script > < script src = "~/lib/SyntaxHighlighter/scripts/shBrushCSharp.js" ></ script > < script src = "~/lib/SyntaxHighlighter/scripts/shBrushDelphi.js" ></ script > < script src = "~/lib/SyntaxHighlighter/scripts/shBrushCpp.js" ></ script > < script src = "~/lib/SyntaxHighlighter/scripts/shBrushDiff.js" ></ script > < script src = "~/lib/SyntaxHighlighter/scripts/shBrushCss.js" ></ script > < script src = "~/lib/SyntaxHighlighter/scripts/shBrushJScript.js" ></ script > < script src = "~/lib/SyntaxHighlighter/scripts/shBrushColdFusion.js" ></ script > < script src = "~/lib/SyntaxHighlighter/scripts/shBrushPerl.js" ></ script > < script src = "~/lib/SyntaxHighlighter/scripts/shBrushJavaFX.js" ></ script > < script src = "~/lib/SyntaxHighlighter/scripts/shBrushGroovy.js" ></ script > < script src = "~/lib/SyntaxHighlighter/scripts/shBrushErlang.js" ></ script > < script src = "~/lib/SyntaxHighlighter/scripts/shBrushJava.js" ></ script > < script src = "~/lib/SyntaxHighlighter/scripts/shBrushPhp.js" ></ script > < script src = "~/lib/SyntaxHighlighter/scripts/shBrushPlain.js" ></ script > < script src = "~/lib/SyntaxHighlighter/scripts/shBrushPowerShell.js" ></ script > < script src = "~/lib/SyntaxHighlighter/scripts/shBrushPython.js" ></ script > < script src = "~/lib/SyntaxHighlighter/scripts/shBrushRuby.js" ></ script > < script src = "~/lib/SyntaxHighlighter/scripts/shBrushSass.js" ></ script > < script src = "~/lib/SyntaxHighlighter/scripts/shBrushScala.js" ></ script > < script src = "~/lib/SyntaxHighlighter/scripts/shBrushSql.js" ></ script > < script src = "~/lib/SyntaxHighlighter/scripts/shBrushVb.js" ></ script > < script src = "~/lib/SyntaxHighlighter/scripts/shBrushXml.js" ></ script > < script src = "~/lib/SyntaxHighlighter/scripts/shLegacy.js" ></ script > < script src = "~/js/site.js" asp-append-version = "true" ></ script > @RenderSection("Scripts", required: false) </ body > </ html > |
_Login.cshtml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 | @model Blog.BAL.Models.LoginViewModel < div class = "modal fade xfooter" id = "login" > < div class = "modal-dialog text-dark" > < div class = "modal-content" > < form asp-controller = "AboutMe" asp-action = "Login" > < div class = "modal-header" > < h5 class = "modal-title" >Login</ h5 > < button type = "button" class = "close" data-dismiss = "modal" aria-label = "Close" > < span aria-hidden = "true" >×</ span > </ button > </ div > < div class = "modal-body" > @if (ViewData.ModelState[""] != null && ViewData.ModelState[""].Errors.Count > 0) { < div class = "alert alert-danger" > < button type = "button" class = "close" data-dismiss = "alert" >×</ button > @Html.ValidationSummary(true, "Errors: ") </ div > } < input name = "IsValid" type = "hidden" value = "@ViewData.ModelState.IsValid.ToString()" /> < div class = "form-group" > < label asp-for = "Password" ></ label > < input type = "password" asp-for = "Password" class = "form-control" /> < span asp-validation-for = "Password" class = "text-danger" ></ span > </ div > < div class = "form-group" > < input type = "checkbox" id = "cbRememberMe" asp-for = "RememberMe" /> < label for = "cbRememberMe" >Remember Me</ label > </ div > </ div > < div class = "modal-footer" > < button type = "button" class = "btn btn-secondary" data-dismiss = "modal" >Close</ button > < button type = "submit" class = "btn btn-primary" data-save = "modal" >Login</ button > </ div > </ form > </ div > </ div > </ div > |